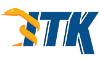 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
22 #include "ITKQuadEdgeMeshExport.h"
30 #define itkQEDebugMacro(x) \
32 std::ostringstream itkmsg; \
33 itkmsg << "Debug: In " __FILE__ ", line " << __LINE__ << '\n' << " (" << this << "): " x << "\n\n"; \
34 OutputWindowDisplayDebugText(itkmsg.str().c_str()); \
36 ITK_MACROEND_NOOP_STATEMENT
37 #define itkQEWarningMacro(x) \
39 std::ostringstream itkmsg; \
40 itkmsg << "WARNING: In " __FILE__ ", line " << __LINE__ << '\n' << " (" << this << "): " x << "\n\n"; \
41 OutputWindowDisplayWarningText(itkmsg.str().c_str()); \
43 ITK_MACROEND_NOOP_STATEMENT
55 #define itkQEAccessorsMacro(st, pt, dt) \
56 pt * GetOnext() { return (dynamic_cast<pt *>(this->st::GetOnext())); } \
58 dt * GetRot() { return (dynamic_cast<dt *>(this->st::GetRot())); } \
60 pt * GetSym() { return (dynamic_cast<pt *>(this->st::GetSym())); } \
62 pt * GetLnext() { return (dynamic_cast<pt *>(this->st::GetLnext())); } \
64 pt * GetRnext() { return (dynamic_cast<pt *>(this->st::GetRnext())); } \
66 pt * GetDnext() { return (dynamic_cast<pt *>(this->st::GetDnext())); } \
68 pt * GetOprev() { return (dynamic_cast<pt *>(this->st::GetOprev())); } \
70 pt * GetLprev() { return (dynamic_cast<pt *>(this->st::GetLprev())); } \
72 pt * GetRprev() { return (dynamic_cast<pt *>(this->st::GetRprev())); } \
74 pt * GetDprev() { return (dynamic_cast<pt *>(this->st::GetDprev())); } \
76 dt * GetInvRot() { return (dynamic_cast<dt *>(this->st::GetInvRot())); } \
78 pt * GetInvOnext() { return (dynamic_cast<pt *>(this->st::GetInvOnext())); } \
80 pt * GetInvLnext() { return (dynamic_cast<pt *>(this->st::GetInvLnext())); } \
82 pt * GetInvRnext() { return (dynamic_cast<pt *>(this->st::GetInvRnext())); } \
84 pt * GetInvDnext() { return (dynamic_cast<pt *>(this->st::GetInvDnext())); } \
85 const pt * GetOnext() const { return (dynamic_cast<const pt *>(this->st::GetOnext())); } \
87 const dt * GetRot() const { return (dynamic_cast<const dt *>(this->st::GetRot())); } \
89 const pt * GetSym() const { return (dynamic_cast<const pt *>(this->st::GetSym())); } \
91 const pt * GetLnext() const { return (dynamic_cast<const pt *>(this->st::GetLnext())); } \
93 const pt * GetRnext() const { return (dynamic_cast<const pt *>(this->st::GetRnext())); } \
95 const pt * GetDnext() const { return (dynamic_cast<const pt *>(this->st::GetDnext())); } \
97 const pt * GetOprev() const { return (dynamic_cast<const pt *>(this->st::GetOprev())); } \
99 const pt * GetLprev() const { return (dynamic_cast<const pt *>(this->st::GetLprev())); } \
101 const pt * GetRprev() const { return (dynamic_cast<const pt *>(this->st::GetRprev())); } \
103 const pt * GetDprev() const { return (dynamic_cast<const pt *>(this->st::GetDprev())); } \
105 const dt * GetInvRot() const { return (dynamic_cast<const dt *>(this->st::GetInvRot())); } \
107 const pt * GetInvOnext() const { return (dynamic_cast<const pt *>(this->st::GetInvOnext())); } \
109 const pt * GetInvLnext() const { return (dynamic_cast<const pt *>(this->st::GetInvLnext())); } \
111 const pt * GetInvRnext() const { return (dynamic_cast<const pt *>(this->st::GetInvRnext())); } \
113 const pt * GetInvDnext() const { return (dynamic_cast<const pt *>(this->st::GetInvDnext())); }
165 operator=(
const QuadEdge &) =
default;
175 this->m_Onext = onext;
190 return this->m_Onext;
200 return this->m_Onext;
230 Self * aNext = this->GetOnext();
238 this->SetOnext(bNext);
253 return (this->m_Rot->m_Rot);
255 return (this->m_Rot);
264 return (this->m_Rot->m_Rot);
266 return (this->m_Rot);
335 return (this->GetRot()->GetRot()->GetRot());
337 Self * p1 = this->GetRot();
360 return this->GetOprev();
365 return this->GetLprev();
370 return this->GetRprev();
375 return this->GetDprev();
381 return (this->GetRot()->GetRot()->GetRot());
383 const Self * p1 = this->GetRot();
405 return this->GetOprev();
410 return this->GetLprev();
415 return this->GetRprev();
420 return this->GetDprev();
427 return ((m_Onext ==
this) || (m_Rot ==
nullptr));
432 return (
this == this->GetOnext());
435 IsEdgeInOnextRing(
Self * testEdge)
const;
439 IsLnextGivenSizeCyclic(
const int size)
const;
const Self * GetInvRnext() const
const Self * GetInvOnext() const
Const iterator for QuadEdgeMesh.
#define itkQEDefineIteratorMethodsMacro(Op)
const Self * GetInvDnext() const
const Self * GetInvLnext() const
void Splice(Self *b)
Basic quad-edge topological method.
const Self * GetOnext() const
void SetOnext(Self *onext)
Base class for the implementation of a quad-edge data structure as proposed in "Guibas and Stolfi 198...
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
const Self * GetRot() const
const Self * GetSym() const
Non const iterator for QuadMesh.
const Self * GetInvRot() const