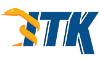 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkRegionConstrainedSubsampler_h
19 #define itkRegionConstrainedSubsampler_h
53 template <
typename TSample,
typename TRegion>
92 itkGetConstReferenceMacro(SampleRegion,
RegionType);
95 itkGetConstReferenceMacro(SampleRegionInitialized,
bool);
100 SetRegionConstraint(
const RegionType & region);
103 itkGetConstReferenceMacro(RegionConstraint,
RegionType);
106 itkGetConstReferenceMacro(RegionConstraintInitialized,
bool);
123 InternalClone()
const override;
129 PrintSelf(std::ostream & os,
Indent indent)
const override;
132 bool m_RegionConstraintInitialized{};
134 bool m_SampleRegionInitialized{};
140 #ifndef ITK_MANUAL_INSTANTIATION
141 # include "itkRegionConstrainedSubsampler.hxx"
SmartPointer< Self > Pointer
SmartPointer< const Self > ConstPointer
ImageBaseType::SizeType SizeType
Control indentation during Print() invocation.
typename SubsampleType::ConstIterator SubsampleConstIterator
std::vector< InstanceIdentifier > InstanceIdentifierHolder
typename SubsampleType::InstanceIdentifierHolder InstanceIdentifierHolder
typename IndexType::IndexValueType IndexValueType
typename TSample::MeasurementVectorType MeasurementVectorType
typename Superclass::Baseclass Baseclass
ImageBaseType::IndexType IndexType
Light weight base class for most itk classes.
typename RegionType::IndexType IndexType
This is the base subsampler class which defines the subsampler API.
typename SubsampleType::Pointer SubsamplePointer
This class stores a subset of instance identifiers from another sample object. You can create a subsa...
typename SampleType::ConstPointer SampleConstPointer
typename RegionType::SizeType SizeType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
This an abstract subsampler that constrains subsamples to be contained within a given image region.
typename TSample::InstanceIdentifier InstanceIdentifier