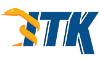 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkScalarImageToRunLengthFeaturesFilter_h
19 #define itkScalarImageToRunLengthFeaturesFilter_h
94 template <
typename TImageType,
typename THistogramFrequencyContainer = DenseFrequencyContainer2>
142 GetFeatureMeansOutput()
const;
145 GetFeatureStandardDeviationsOutput()
const;
149 using Superclass::SetInput;
175 SetNumberOfBinsPerAxis(
unsigned int);
186 SetDistanceValueMinMax(
double min,
double max);
195 GetMaskImage()
const;
200 SetInsidePixelValue(
PixelType insidePixelValue);
202 itkGetConstMacro(FastCalculations,
bool);
203 itkSetMacro(FastCalculations,
bool);
204 itkBooleanMacro(FastCalculations);
210 PrintSelf(std::ostream & os,
Indent indent)
const override;
220 GenerateData()
override;
224 using Superclass::MakeOutput;
234 bool m_FastCalculations{};
239 #ifndef ITK_MANUAL_INSTANTIATION
240 # include "itkScalarImageToRunLengthFeaturesFilter.hxx"
SmartPointer< Self > Pointer
This class computes a run length matrix (histogram) from a given image and a mask image if provided....
SmartPointer< const Self > ConstPointer
Define a front-end to the STL "vector" container that conforms to the IndexedContainerInterface.
uint8_t RunLengthFeatureName
This class computes texture feature coefficients from a grey level run-length matrix.
This class computes run length descriptions from an image.
typename ImageType::PixelType PixelType
Control indentation during Print() invocation.
DataObjectPointerArray::size_type DataObjectPointerArraySizeType
typename FeatureValueVector::Pointer FeatureValueVectorPointer
Decorates any subclass of itkObject with a DataObject API.
This class stores measurement vectors in the context of n-dimensional histogram.
typename ImageType::Pointer ImagePointer
typename ImageType::OffsetType OffsetType
Light weight base class for most itk classes.
typename RunLengthMatrixFilterType::HistogramType HistogramType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename FeatureNameVector::ConstPointer FeatureNameVectorConstPointer
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
class ITK_FORWARD_EXPORT ProcessObject
typename FeatureNameVector::Pointer FeatureNameVectorPointer
typename OffsetVector::ConstPointer OffsetVectorConstPointer
SmartPointer< Self > Pointer
THistogramFrequencyContainer FrequencyContainerType
typename OffsetVector::Pointer OffsetVectorPointer