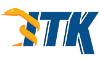 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkScalarImageToTextureFeaturesFilter_h
19 #define itkScalarImageToTextureFeaturesFilter_h
108 template <
typename TImageType,
109 typename THistogramFrequencyContainer = DenseFrequencyContainer2,
110 typename TMaskImageType = TImageType>
162 GetFeatureMeansOutput()
const;
165 GetFeatureStandardDeviationsOutput()
const;
169 using Superclass::SetInput;
195 SetNumberOfBinsPerAxis(
unsigned int);
209 GetMaskImage()
const;
216 itkGetConstMacro(FastCalculations,
bool);
217 itkSetMacro(FastCalculations,
bool);
218 itkBooleanMacro(FastCalculations);
224 PrintSelf(std::ostream & os,
Indent indent)
const override;
234 GenerateData()
override;
238 using Superclass::MakeOutput;
250 bool m_FastCalculations{};
255 #ifndef ITK_MANUAL_INSTANTIATION
256 # include "itkScalarImageToTextureFeaturesFilter.hxx"
SmartPointer< Self > Pointer
typename FeatureNameVector::Pointer FeatureNameVectorPointer
SmartPointer< const Self > ConstPointer
Define a front-end to the STL "vector" container that conforms to the IndexedContainerInterface.
typename OffsetVector::Pointer OffsetVectorPointer
typename ImageType::Pointer ImagePointer
TMaskImageType MaskImageType
uint8_t TextureFeatureName
Control indentation during Print() invocation.
DataObjectPointerArray::size_type DataObjectPointerArraySizeType
typename MaskImageType::Pointer MaskPointer
Decorates any subclass of itkObject with a DataObject API.
This class computes a co-occurrence matrix (histogram) from a given image and a mask image if provide...
This class stores measurement vectors in the context of n-dimensional histogram.
Light weight base class for most itk classes.
This class computes texture feature coefficients from a grey level co-occurrence matrix.
typename MaskImageType::PixelType MaskPixelType
typename FeatureNameVector::ConstPointer FeatureNameVectorConstPointer
THistogramFrequencyContainer FrequencyContainerType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
typename CooccurrenceMatrixFilterType::HistogramType HistogramType
class ITK_FORWARD_EXPORT ProcessObject
typename ImageType::OffsetType OffsetType
This class computes texture descriptions from an image.
typename FeatureValueVector::Pointer FeatureValueVectorPointer
typename OffsetVector::ConstPointer OffsetVectorConstPointer
SmartPointer< Self > Pointer
typename ImageType::PixelType PixelType