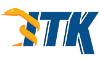 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
19 #ifndef itkShapedImageNeighborhoodRange_h
20 #define itkShapedImageNeighborhoodRange_h
27 #include <type_traits>
90 template <
typename TImage,
91 typename TImageNeighborhoodPixelAccessPolicy = ZeroFluxNeumannImageNeighborhoodPixelAccessPolicy<TImage>>
108 template <
typename T>
110 Test(
typename T::PixelAccessParameterType *);
112 template <
typename T>
119 !std::is_same_v<decltype(Test<TImageNeighborhoodPixelAccessPolicy>(
nullptr)),
120 decltype(Test<TImageNeighborhoodPixelAccessPolicy>())>;
124 template <
typename TPolicy,
bool VPolicyHasPixelAccessParameterType = CheckPolicy::HasPixelAccessParameterType>
127 using Type =
typename TPolicy::PixelAccessParameterType;
131 template <
typename TPolicy>
161 template <
bool VIsConst,
typename TDummy =
void>
167 template <
typename TDummy>
189 const TImageNeighborhoodPixelAccessPolicy & pixelAccessPolicy) noexcept
191 , m_PixelAccessPolicy{ pixelAccessPolicy }
197 , m_PixelAccessPolicy{ pixelProxy.m_PixelAccessPolicy }
207 template <
typename TDummy>
231 const TImageNeighborhoodPixelAccessPolicy & pixelAccessPolicy) noexcept
233 , m_PixelAccessPolicy{ pixelAccessPolicy }
262 const auto lhsPixelValue = lhs.m_PixelAccessPolicy.GetPixelValue(lhs.m_ImageBufferPointer);
263 const auto rhsPixelValue = rhs.m_PixelAccessPolicy.GetPixelValue(rhs.m_ImageBufferPointer);
266 lhs.m_PixelAccessPolicy.SetPixelValue(lhs.m_ImageBufferPointer, rhsPixelValue);
267 rhs.m_PixelAccessPolicy.SetPixelValue(rhs.m_ImageBufferPointer, lhsPixelValue);
284 template <
bool VIsConst>
352 return TImageNeighborhoodPixelAccessPolicy{
357 template <
typename TPixelAccessParameter>
358 TImageNeighborhoodPixelAccessPolicy
361 static_assert(std::is_same_v<TPixelAccessParameter, OptionalPixelAccessParameterType>,
362 "This helper function should only be used for OptionalPixelAccessParameterType!");
363 static_assert(!std::is_same_v<TPixelAccessParameter, EmptyPixelAccessParameter>,
364 "EmptyPixelAccessParameter indicates that there is no pixel access parameter specified!");
365 return TImageNeighborhoodPixelAccessPolicy{
390 template <
bool VIsArgumentConst,
typename = std::enable_if_t<VIsConst && !VIsArgumentConst>>
466 assert(lhs.m_ImageBufferPointer == rhs.m_ImageBufferPointer);
467 assert(lhs.m_ImageSize == rhs.m_ImageSize);
468 assert(lhs.m_OffsetTable == rhs.m_OffsetTable);
471 return lhs.m_CurrentOffset == rhs.m_CurrentOffset;
480 return !(lhs == rhs);
488 assert(lhs.m_ImageBufferPointer == rhs.m_ImageBufferPointer);
489 assert(lhs.m_ImageSize == rhs.m_ImageSize);
490 assert(lhs.m_OffsetTable == rhs.m_OffsetTable);
493 return lhs.m_CurrentOffset < rhs.m_CurrentOffset;
546 assert(lhs.m_ImageBufferPointer == rhs.m_ImageBufferPointer);
547 assert(lhs.m_ImageSize == rhs.m_ImageSize);
548 assert(lhs.m_OffsetTable == rhs.m_OffsetTable);
551 return lhs.m_CurrentOffset - rhs.m_CurrentOffset;
597 :
m_Index(imageRegion.GetIndex())
598 ,
m_Size(imageRegion.GetSize())
608 index1[i] -= index2[i];
661 const size_t numberOfNeigborhoodPixels,
676 assert(offsetTable !=
nullptr);
694 template <
typename TContainerOfOffsets>
697 const TContainerOfOffsets & shapeOffsets,
701 std::data(shapeOffsets),
702 std::size(shapeOffsets),
703 optionalPixelAccessParameter }
733 return this->
begin();
795 assert(n < this->
size());
796 assert(n <= static_cast<size_t>(std::numeric_limits<ptrdiff_t>::max()));
799 return this->
begin()[static_cast<ptrdiff_t>(n)];
QualifiedIterator(QualifiedInternalPixelType *const imageBufferPointer, const ImageSizeType &imageSize, const OffsetType &offsetTable, const NeighborhoodAccessorFunctorType &neighborhoodAccessor, const OptionalPixelAccessParameterType optionalPixelAccessParameter, const IndexType &relativeLocation, const OffsetType *const offset) noexcept
const_iterator cend() const noexcept
QualifiedIterator< false >::reference operator[](const vcl_size_t n) const noexcept
RegionData m_BufferedRegionData
const_reverse_iterator crbegin() const noexcept
typename TImage::IndexValueType IndexValueType
QualifiedIterator(const QualifiedIterator< VIsArgumentConst > &arg) noexcept
PixelProxy(InternalPixelType *const imageBufferPointer, const TImageNeighborhoodPixelAccessPolicy &pixelAccessPolicy) noexcept
typename TImage::SizeType ImageSizeType
constexpr iterator begin()
static constexpr bool IsImageTypeConst
QualifiedIterator operator++(int) noexcept
const_reverse_iterator crend() const noexcept
QualifiedPixelType * pointer
PixelProxy & operator=(const PixelType &pixelValue) noexcept
std::conditional_t< IsImageTypeConst, const PixelType, PixelType > QualifiedPixelType
std::conditional_t< IsImageTypeConst, const InternalPixelType, InternalPixelType > QualifiedInternalPixelType
QualifiedIterator operator--(int) noexcept
iterator begin() const noexcept
IndexType m_RelativeLocation
friend bool operator==(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
typename TImage::RegionType ImageRegionType
QualifiedIterator< true > const_iterator
friend QualifiedIterator operator+(const difference_type n, QualifiedIterator it) noexcept
NeighborhoodAccessorFunctorType m_NeighborhoodAccessor
typename TImage::PixelType PixelType
friend bool operator!=(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
ImageBaseType::SizeType SizeType
void SetLocation(const IndexType &location) noexcept
InternalPixelType *const m_ImageBufferPointer
std::reverse_iterator< iterator > reverse_iterator
PixelProxy(const PixelProxy< false > &pixelProxy) noexcept
QualifiedIterator()=default
const_iterator cbegin() const noexcept
static int Test(typename T::PixelAccessParameterType *)
friend difference_type operator-(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
OptionalPixelAccessParameterType m_OptionalPixelAccessParameter
friend bool operator<(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
friend QualifiedIterator operator-(QualifiedIterator it, const difference_type n) noexcept
friend void swap(PixelProxy lhs, PixelProxy rhs) noexcept
ImageBaseType::IndexType IndexType
const TImageNeighborhoodPixelAccessPolicy m_PixelAccessPolicy
static constexpr bool HasPixelAccessParameterType
IndexType m_RelativeLocation
const TImageNeighborhoodPixelAccessPolicy m_PixelAccessPolicy
OptionalPixelAccessParameterType m_OptionalPixelAccessParameter
reference operator*() const noexcept
PixelProxy & operator=(const PixelProxy &pixelProxy) noexcept
const InternalPixelType *const m_ImageBufferPointer
const OffsetType * m_ShapeOffsets
friend QualifiedIterator & operator+=(QualifiedIterator &it, const difference_type n) noexcept
friend bool operator>(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
typename TImage::NeighborhoodAccessorFunctorType NeighborhoodAccessorFunctorType
typename TImage::SizeValueType ImageSizeValueType
QualifiedInternalPixelType * m_ImageBufferPointer
static constexpr bool IsImageTypeConst
ImageBaseType::RegionType RegionType
QualifiedIterator & operator--() noexcept
ptrdiff_t difference_type
RegionData() noexcept=default
vcl_size_t size() const noexcept
friend bool operator<=(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
reverse_iterator rend() const noexcept
QualifiedIterator & operator++() noexcept
QualifiedInternalPixelType * m_ImageBufferPointer
std::reverse_iterator< const_iterator > const_reverse_iterator
ImageSizeType m_ImageSize
void SubtractIndex(IndexType &index1, const IndexType &index2)
std::conditional_t< IsImageTypeConst, const InternalPixelType, InternalPixelType > QualifiedInternalPixelType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
friend bool operator>=(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
QualifiedIterator< IsImageTypeConst > iterator
friend QualifiedIterator & operator-=(QualifiedIterator &it, const difference_type n) noexcept
ShapedImageNeighborhoodRange(ImageType &image, const IndexType &location, const TContainerOfOffsets &shapeOffsets, const OptionalPixelAccessParameterType optionalPixelAccessParameter={})
bool empty() const noexcept
ShapedImageNeighborhoodRange()=default
typename OptionalPixelAccessParameter< TImageNeighborhoodPixelAccessPolicy >::Type OptionalPixelAccessParameterType
TImageNeighborhoodPixelAccessPolicy CreatePixelAccessPolicy(EmptyPixelAccessParameter) const
TImageNeighborhoodPixelAccessPolicy CreatePixelAccessPolicy(const TPixelAccessParameter pixelAccessParameter) const
std::random_access_iterator_tag iterator_category
NeighborhoodAccessorFunctorType m_NeighborhoodAccessor
typename TImage::ImageDimensionType ImageDimensionType
typename TImage::IndexType IndexType
reverse_iterator rbegin() const noexcept
iterator end() const noexcept
reference operator[](const difference_type n) const noexcept
PixelProxy(const InternalPixelType *const imageBufferPointer, const TImageNeighborhoodPixelAccessPolicy &pixelAccessPolicy) noexcept
std::conditional_t< VIsConst, const ImageType, ImageType > QualifiedImageType
const OffsetType * m_CurrentOffset
vcl_size_t m_NumberOfNeighborhoodPixels
static constexpr ImageDimensionType ImageDimension
friend QualifiedIterator operator+(QualifiedIterator it, const difference_type n) noexcept
typename TImageNeighborhoodPixelAccessPolicy ::PixelAccessParameterType Type
typename TImage::InternalPixelType InternalPixelType
unsigned long SizeValueType
ShapedImageNeighborhoodRange(ImageType &image, const IndexType &location, const OffsetType *const shapeOffsets, const vcl_size_t numberOfNeigborhoodPixels, const OptionalPixelAccessParameterType optionalPixelAccessParameter={})