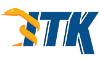 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkSpecialCoordinatesImage_h
19 #define itkSpecialCoordinatesImage_h
94 template <
typename TPixel,
unsigned int VImageDimension = 2>
141 static constexpr
unsigned int ImageDimension = VImageDimension;
147 using typename Superclass::OffsetType;
163 using typename Superclass::SpacingType;
176 Allocate(
bool initialize =
false)
override;
181 Initialize()
override;
186 FillBuffer(
const TPixel & value);
197 (*m_Buffer)[offset] = value;
208 return ((*m_Buffer)[offset]);
219 return ((*m_Buffer)[offset]);
239 return m_Buffer ? m_Buffer->GetBufferPointer() :
nullptr;
244 return m_Buffer ? m_Buffer->GetBufferPointer() :
nullptr;
252 return m_Buffer.GetPointer();
255 const PixelContainer *
258 return m_Buffer.GetPointer();
264 SetPixelContainer(PixelContainer * container);
332 PrintSelf(std::ostream & os,
Indent indent)
const override;
342 #ifndef ITK_MANUAL_INSTANTIATION
343 # include "itkSpecialCoordinatesImage.hxx"
TPixel & operator[](const IndexType &index)
Access a pixel. This version can be an lvalue.
typename PixelContainer::Pointer PixelContainerPointer
typename OffsetType::OffsetValueType OffsetValueType
SmartPointer< Self > Pointer
AccessorType GetPixelAccessor()
SmartPointer< const Self > ConstPointer
Base class for templated image classes.
PixelContainer * GetPixelContainer()
ImageBaseType::PointType PointType
void SetSpacing(const double[VImageDimension]) override
ImageBaseType::SizeType SizeType
const AccessorType GetPixelAccessor() const
Give access to partial aspects a type.
Control indentation during Print() invocation.
Templated n-dimensional nonrectilinear-coordinate image base class.
ImageBaseType::IndexType IndexType
Provides a common API for pixel accessors for Image and VectorImage.
void SetSpacing(const SpacingType &) override
const TPixel & operator[](const IndexType &index) const
Access a pixel. This version can only be an rvalue.
ImageBaseType::RegionType RegionType
const TPixel * GetBufferPointer() const
typename PixelContainer::ConstPointer PixelContainerConstPointer
TPixel & GetPixel(const IndexType &index)
Get a reference to a pixel (e.g. for editing).
const PixelContainer * GetPixelContainer() const
Defines an itk::Image front-end to a standard C-array.
TPixel * GetBufferPointer()
Implements a weak reference to an object.
void SetOrigin(const float[VImageDimension]) override
void SetPixel(const IndexType &index, const TPixel &value)
Set a pixel value.
void SetSpacing(const float[VImageDimension]) override
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
void SetOrigin(const PointType) override
Base class for most ITK classes.
void SetOrigin(const double[VImageDimension]) override
const TPixel & GetPixel(const IndexType &index) const
Get a pixel (read only version).
Base class for all data objects in ITK.