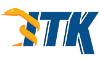 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkStatisticsLabelObjectAccessors_h
19 #define itkStatisticsLabelObjectAccessors_h
35 template <
typename TLabelObject>
45 return labelObject->GetMinimum();
49 template <
typename TLabelObject>
59 return labelObject->GetMaximum();
63 template <
typename TLabelObject>
73 return labelObject->GetMean();
77 template <
typename TLabelObject>
87 return labelObject->GetSum();
91 template <
typename TLabelObject>
101 return labelObject->GetStandardDeviation();
105 template <
typename TLabelObject>
115 return labelObject->GetVariance();
119 template <
typename TLabelObject>
129 return labelObject->GetMedian();
133 template <
typename TLabelObject>
143 return labelObject->GetMaximumIndex();
147 template <
typename TLabelObject>
157 return labelObject->GetMinimumIndex();
161 template <
typename TLabelObject>
171 return labelObject->GetCenterOfGravity();
190 template <
typename TLabelObject>
200 return labelObject->GetWeightedPrincipalMoments();
204 template <
typename TLabelObject>
214 return labelObject->GetWeightedPrincipalAxes();
218 template <
typename TLabelObject>
228 return labelObject->GetKurtosis();
232 template <
typename TLabelObject>
242 return labelObject->GetSkewness();
246 template <
typename TLabelObject>
256 return labelObject->GetWeightedElongation();
260 template <
typename TLabelObject>
271 return labelObject->GetHistogram();
275 template <
typename TLabelObject>
285 return labelObject->GetWeightedFlatness();
typename LabelObjectType::IndexType AttributeValueType
TLabelObject LabelObjectType
TLabelObject LabelObjectType
typename LabelObjectType::MatrixType AttributeValueType
typename LabelObjectType::IndexType AttributeValueType
double AttributeValueType
double AttributeValueType
ImageBaseType::SpacingType VectorType
ImageBaseType::PointType PointType
TLabelObject LabelObjectType
TLabelObject LabelObjectType
AttributeValueType operator()(const LabelObjectType *labelObject) const
TLabelObject LabelObjectType
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
double AttributeValueType
double AttributeValueType
double AttributeValueType
TLabelObject LabelObjectType
AttributeValueType operator()(const LabelObjectType *labelObject) const
typename LabelObjectType::HistogramType * AttributeValueType
TLabelObject LabelObjectType
double AttributeValueType
AttributeValueType operator()(const LabelObjectType *labelObject) const
ImageBaseType::IndexType IndexType
double AttributeValueType
TLabelObject LabelObjectType
AttributeValueType operator()(const LabelObjectType *labelObject) const
double AttributeValueType
TLabelObject LabelObjectType
AttributeValueType operator()(const LabelObjectType *labelObject) const
typename LabelObjectType::PointType AttributeValueType
TLabelObject LabelObjectType
TLabelObject LabelObjectType
double AttributeValueType
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
TLabelObject LabelObjectType
TLabelObject LabelObjectType
TLabelObject LabelObjectType
TLabelObject LabelObjectType
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
AttributeValueType operator()(const LabelObjectType *labelObject) const
TLabelObject LabelObjectType
double AttributeValueType
typename LabelObjectType::VectorType AttributeValueType
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const