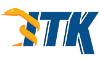 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkTestingHashImageFilter_h
19 #define itkTestingHashImageFilter_h
64 template <
typename TImageType>
94 return this->GetHashOutput()->Get();
101 const HashObjectType *
111 using Superclass::MakeOutput;
116 #if !defined(ITK_LEGACY_REMOVE)
134 PrintSelf(std::ostream & os,
Indent indent)
const override;
150 AfterThreadedGenerateData()
override;
156 EnlargeOutputRequestedRegion(
DataObject * data)
override;
166 #include "itkTestingHashImageFilter.hxx"
168 #endif // itkTestingHashImageFilter_h
Generates a md5 hash string from an image.
Decorates any "simple" data type (data types without smart pointers) with a DataObject API.
const HashObjectType * GetHashOutput() const
Base class for filters that take an image as input and overwrite that image as the output.
DataObject * GetOutput(const DataObjectIdentifierType &key)
Control indentation during Print() invocation.
DataObjectPointerArray::size_type DataObjectPointerArraySizeType
void ThreadedGenerateData(const typename Superclass::OutputImageRegionType &, ThreadIdType) override
unsigned int ThreadIdType
Light weight base class for most itk classes.
HashObjectType * GetHashOutput()
ImageBaseType::RegionType RegionType
void DynamicThreadedGenerateData(const typename Superclass::OutputImageRegionType &) override
std::ostream & operator<<(std::ostream &out, const ExtractSliceImageFilterEnums::TestExtractSliceImageFilterCollapseStrategy value)
Enum classes for HashImageFilter.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
std::string GetHash() const
SmartPointer< Self > Pointer
typename TImageType::RegionType RegionType
Base class for all data objects in ITK.