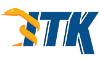 |
ITK
5.1.0
Insight Toolkit
|
Go to the documentation of this file.
42 template <
typename TValue>
46 ITK_DISALLOW_COPY_AND_ASSIGN(
TreeNode);
68 Set(
const TValue data);
92 CountChildren()
const;
100 GetNumberOfChildren(
unsigned int depth = 0,
char * name =
nullptr)
const;
104 ReplaceChild(
Self * oldChild,
Self * newChild);
108 ChildPosition(
const Self * node)
const;
112 ChildPosition(TValue node)
const;
116 AddChild(
Self * node);
123 #if !defined(ITK_WRAPPING_PARSER)
125 GetChildren(
unsigned int depth = 0,
char * name =
nullptr)
const;
130 #if !defined(ITK_WRAPPING_PARSER)
152 #ifndef ITK_MANUAL_INSTANTIATION
153 # include "itkTreeNode.hxx"
Represents a node in a tree.
::itk::OffsetValueType ChildIdentifier
Light weight base class for most itk classes.
std::vector< Pointer > ChildrenListType
virtual ChildrenListType & GetChildrenList()
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
signed long OffsetValueType
Base class for most ITK classes.
ChildrenListType m_Children