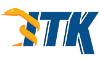 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkTriangleMeshToSimplexMeshFilter_h
19 #define itkTriangleMeshToSimplexMeshFilter_h
44 template <
typename TInputMesh,
typename TOutputMesh>
121 PrintSelf(std::ostream & os,
Indent indent)
const override;
127 GenerateData()
override;
139 CreateSimplexPoints();
154 CreateSimplexNeighbors();
239 #ifndef ITK_MANUAL_INSTANTIATION
240 # include "itkTriangleMeshToSimplexMeshFilter.hxx"
typename TOutputMesh::PointIdentifier PointIdentifier
SmartPointer< Self > Pointer
typename TInputMesh::CellType InputCellType
Represents a single vertex for a Mesh.
Define a front-end to the STL "vector" container that conforms to the IndexedContainerInterface.
This filter converts a triangle mesh into a 2-simplex mesh.
typename InputPointsContainer::Pointer InputPointsContainerPointer
typename OutputMeshType::Pointer OutputMeshPointer
typename TOutputMesh::PointsContainer OutputPointsContainer
typename TOutputMesh::CellAutoPointer OutputCellAutoPointer
typename InputMeshType::Pointer InputMeshPointer
ImageBaseType::PointType PointType
typename EdgeMapType::Pointer EdgeMapPointer
typename OutputPointsContainer::Pointer OutputPointsContainerPointer
typename TInputMesh::BoundaryAssignmentsContainerPointer InputBoundaryAssignmentsContainerPointer
typename TOutputMesh::PointType OutputPointType
std::pair< CellIdentifier, CellIdentifier > EdgeIdentifierType
Represents a polygon in a Mesh.
Control indentation during Print() invocation.
typename TOutputMesh::PixelType OutputPixelType
typename TOutputMesh::CellType OutputCellType
typename TOutputMesh::CellFeatureIdentifier CellFeatureIdentifier
A wrapper of the STL "map" container.
typename TOutputMesh::CellIdentifier CellIdentifier
Light weight base class for most itk classes.
typename TOutputMesh::PointsContainer::Iterator OutputPointsContainerIterator
MeshToMeshFilter is the base class for all process objects that output mesh data, and require mesh da...
typename InputPointsContainer::ConstIterator InputPointsContainerConstIterator
typename IdVectorType::Pointer IdVectorPointer
typename TInputMesh::PointsContainer InputPointsContainer
Represents a line segment for a Mesh.
typename TInputMesh::CellAutoPointer CellAutoPointer
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename TInputMesh::PointType InputPointType
std::set< CellIdentifier > IndexSetType
typename TInputMesh::BoundaryAssignmentIdentifier InputBoundnaryAssignmentIdentifier
typename InputCellType::CellAutoPointer InputCellAutoPointer
typename InputPointsContainer::Iterator InputPointsContainerIterator