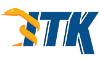 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkVectorImageNeighborhoodAccessorFunctor_h
19 #define itkVectorImageNeighborhoodAccessorFunctor_h
40 template <
typename TImage>
52 template <
typename TOutput = ImageType>
77 this->
m_Begin = const_cast<InternalPixelType *>(begin);
102 truePixelPointer[i] = p[i];
107 template <
typename TOutput>
114 return boundaryCondition->operator()(point_index, boundary_offset, data, *
this);
typename TOutputImage::PixelType OutputPixelType
void Set(InternalPixelType *const pixelPointer, const PixelType &p) const
VectorImageNeighborhoodAccessorFunctor()=default
unsigned int VectorLengthType
A light-weight container object for storing an N-dimensional neighborhood of values.
typename ImageType::InternalPixelType InternalPixelType
VectorLengthType GetVectorLength()
void SetBegin(const InternalPixelType *begin)
ImageBoundaryConditionType< TOutput >::OutputPixelType BoundaryCondition(const OffsetType &point_index, const OffsetType &boundary_offset, const NeighborhoodType *data, const ImageBoundaryConditionType< TOutput > *boundaryCondition) const
A virtual base object that defines an interface to a class of boundary condition objects for use by n...
VectorImageNeighborhoodAccessorFunctor(VectorLengthType length)
InternalPixelType * m_Begin
void SetVectorLength(VectorLengthType length)
VectorLengthType m_OffsetMultiplier
typename ImageType::PixelType PixelType
PixelType Get(const InternalPixelType *pixelPointer) const
Provides accessor interfaces to Access pixels and is meant to be used on pointers to pixels held by t...
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename ImageType::OffsetType OffsetType
VectorLengthType m_VectorLength