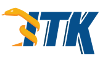 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkArithmeticOpsFunctors_h
19 #define itkArithmeticOpsFunctors_h
33 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
34 class ITK_TEMPLATE_EXPORT
Add2
49 return !(*
this != other);
55 return static_cast<TOutput>(A + B);
65 template <
typename TInput1,
typename TInput2,
typename TInput3,
typename TOutput>
66 class ITK_TEMPLATE_EXPORT
Add3
81 return !(*
this != other);
85 operator()(
const TInput1 & A,
const TInput2 & B,
const TInput3 & C)
const
87 return static_cast<TOutput>(A + B + C);
97 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
98 class ITK_TEMPLATE_EXPORT
Sub2
113 return !(*
this != other);
119 return static_cast<TOutput>(A - B);
129 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
145 return !(*
this != other);
151 return static_cast<TOutput>(A * B);
161 template <
typename TInput1,
typename TInput2,
typename TOutput>
162 class ITK_TEMPLATE_EXPORT
Div
177 return !(*
this != other);
185 return (TOutput)(A / B);
200 template <
typename TNumerator,
typename TDenominator = TNumerator,
typename TOutput = TNumerator>
216 return !(*
this == other);
228 operator()(
const TNumerator & n,
const TDenominator & d)
const
234 return static_cast<TOutput>(n) / static_cast<TOutput>(d);
246 template <
typename TInput1,
typename TInput2,
typename TOutput>
263 return !(*
this != other);
271 return static_cast<TOutput>(A % B);
280 #if !defined(ITK_FUTURE_LEGACY_REMOVE)
290 template <
typename TInput,
typename TOutput>
291 class ITK_TEMPLATE_EXPORT ModulusTransform
294 ModulusTransform() { m_Dividend = 5; }
295 ~ModulusTransform() =
default;
297 SetDividend(TOutput dividend)
299 m_Dividend = dividend;
304 operator!=(
const ModulusTransform & other)
const
306 if (m_Dividend != other.m_Dividend)
314 operator==(
const ModulusTransform & other)
const
316 return !(*
this != other);
320 operator()(
const TInput & x)
const
322 auto result = static_cast<TOutput>(x % m_Dividend);
342 template <
class TInput1,
class TInput2,
class TOutput>
355 return !(*
this != other);
361 const double temp = std::floor(
double(A) /
double(B));
373 return static_cast<TOutput>(temp);
388 template <
class TInput1,
class TInput2,
class TOutput>
402 return !(*
this != other);
419 template <
class TInput1,
class TOutput = TInput1>
435 return !(*
this != other);
441 return (TOutput)(-A);
TOutput operator()(const TInput1 &A, const TInput2 &B) const
TOutput operator()(const TInput1 &A) const
bool operator==(const DivReal &other) const
bool operator==(const Mult &other) const
bool operator!=(const UnaryMinus &) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const
bool operator==(const UnaryMinus &other) const
bool operator==(const Add3 &other) const
static constexpr T NonpositiveMin()
bool operator!=(const Sub2 &) const
bool operator==(const DivideOrZeroOut &) const
bool operator!=(const Add2 &) const
bool operator!=(const DivFloor &) const
bool operator!=(const Modulus &) const
TOutput operator()(const TNumerator &n, const TDenominator &d) const
bool operator==(const Modulus &other) const
bool operator!=(const Add3 &) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const
TOutput operator()(const TInput1 &A, const TInput2 &B, const TInput3 &C) const
bool NotAlmostEquals(T1 x1, T2 x2)
bool operator==(const Add2 &other) const
bool operator==(const Div &other) const
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
TOutput operator()(const TInput1 &A, const TInput2 &B) const
Cast arguments to double, performs division then takes the floor.
TOutput operator()(const TInput1 &A, const TInput2 &B) const
Define additional traits for native types such as int or float.
static constexpr T max(const T &)
bool operator!=(const Index< VDimension > &one, const Index< VDimension > &two)
bool operator!=(const Mult &) const
bool operator==(const DivFloor &other) const
Promotes arguments to real type and performs division.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Apply the unary minus operator.
bool operator!=(const Div &) const
static constexpr double e
bool operator!=(const DivideOrZeroOut &other) const
bool operator==(const Sub2 &other) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const
bool operator!=(const DivReal &) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const