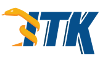 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
65 template <
unsigned int VDimension = 2>
66 struct ITK_TEMPLATE_EXPORT
Index final
87 static constexpr
unsigned int Dimension = VDimension;
90 static constexpr
unsigned int
103 for (
unsigned int i = 0; i < VDimension; i++)
105 result[i] = m_InternalArray[i] + static_cast<IndexValueType>(sz[i]);
114 for (
unsigned int i = 0; i < VDimension; i++)
116 m_InternalArray[i] += static_cast<IndexValueType>(sz[i]);
129 for (
unsigned int i = 0; i < VDimension; i++)
131 result[i] = m_InternalArray[i] - static_cast<IndexValueType>(sz[i]);
140 for (
unsigned int i = 0; i < VDimension; i++)
142 m_InternalArray[i] -= static_cast<IndexValueType>(sz[i]);
154 for (
unsigned int i = 0; i < VDimension; i++)
156 result[i] = m_InternalArray[i] + offset[i];
165 for (
unsigned int i = 0; i < VDimension; i++)
167 m_InternalArray[i] += offset[i];
177 for (
unsigned int i = 0; i < VDimension; i++)
179 m_InternalArray[i] -= offset[i];
191 for (
unsigned int i = 0; i < VDimension; i++)
204 for (
unsigned int i = 0; i < VDimension; i++)
218 for (
unsigned int i = 0; i < VDimension; i++)
220 result[i] = m_InternalArray[i] * static_cast<IndexValueType>(vec.
m_InternalArray[i]);
230 return m_InternalArray;
240 std::copy_n(val, VDimension, m_InternalArray);
252 m_InternalArray[element] = val;
264 return m_InternalArray[element];
272 std::fill_n(begin(), size(), value);
289 static_assert(VDimension > 0,
"Error: Only positive value sized VDimension allowed");
294 template <
typename TCoordRep>
298 for (
unsigned int i = 0; i < VDimension; ++i)
300 m_InternalArray[i] = Math::Round<IndexValueType>(point[i]);
306 template <
typename TCoordRep>
310 for (
unsigned int i = 0; i < VDimension; ++i)
312 m_InternalArray[i] = static_cast<IndexValueType>(point[i]);
321 GetBasisIndex(
unsigned int dim);
347 std::fill_n(begin(), size(), newValue);
359 return iterator(&m_InternalArray[0]);
371 return iterator(&m_InternalArray[VDimension]);
386 const_reverse_iterator
398 const_reverse_iterator
429 ExceptionThrowingBoundsCheck(pos);
430 return m_InternalArray[pos];
436 ExceptionThrowingBoundsCheck(pos);
437 return m_InternalArray[pos];
455 return VDimension ? *(end() - 1) : *end();
461 return VDimension ? *(end() - 1) : *end();
467 return &m_InternalArray[0];
473 return &m_InternalArray[0];
493 if (pos >= VDimension)
495 throw std::out_of_range(
"array::ExceptionThrowingBoundsCheck");
501 template <
unsigned int VDimension>
511 template <
unsigned int VDimension>
516 for (
unsigned int i = 0; i + 1 < VDimension; ++i)
518 os << obj[i] <<
", ";
522 os << obj[VDimension - 1];
530 template <
unsigned int VDimension>
537 template <
unsigned int VDimension>
541 return !(one == two);
544 template <
unsigned int VDimension>
548 return std::lexicographical_compare(one.
begin(), one.
end(), two.
begin(), two.
end());
551 template <
unsigned int VDimension>
558 template <
unsigned int VDimension>
565 template <
unsigned int VDimension>
573 template <
unsigned int VDimension>
581 template <
unsigned int VDimension>
std::reverse_iterator< const_iterator > const_reverse_iterator
Represent a n-dimensional index in a n-dimensional image.
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
bool operator<=(const Index< VDimension > &one, const Index< VDimension > &two)
constexpr size_type max_size() const
Represent a n-dimensional size (bounds) of a n-dimensional image.
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
void assign(const value_type &newValue)
void CopyWithRound(const FixedArray< TCoordRep, VDimension > &point)
const_reference operator[](size_type pos) const
void swap(Array< T > &a, Array< T > &b)
::itk::IndexValueType IndexValueType
const_reference at(size_type pos) const
void SetIndex(const IndexValueType val[VDimension])
const Self & operator+=(const OffsetType &offset)
const Self operator*(const SizeType &vec) const
static constexpr unsigned int Dimension
void swap(Index< VDimension > &one, Index< VDimension > &two)
const Self operator-(const OffsetType &off) const
void SetElement(unsigned long element, IndexValueType val)
const Self operator+(const SizeType &sz) const
void Fill(IndexValueType value)
const_reference front() const
const_reference back() const
const Self operator+(const OffsetType &offset) const
std::ptrdiff_t difference_type
const_iterator begin() const
reference operator[](size_type pos)
const IndexValueType * GetIndex() const
const_reverse_iterator rend() const
::itk::OffsetValueType OffsetValueType
reference at(size_type pos)
constexpr size_type size() const
const OffsetType operator-(const Self &vec) const
const value_type & const_reference
IndexValueType m_InternalArray[VDimension]
bool operator>=(const Index< VDimension > &one, const Index< VDimension > &two)
OffsetValueType m_InternalArray[VDimension]
const_reverse_iterator rbegin() const
bool operator>(const Index< VDimension > &one, const Index< VDimension > &two)
constexpr bool empty() const
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
Simulate a standard C array with copy semantics.
void CopyWithCast(const FixedArray< TCoordRep, VDimension > &point)
static constexpr unsigned int GetIndexDimension()
IndexValueType GetElement(unsigned long element) const
Represent a n-dimensional offset between two n-dimensional indexes of n-dimensional image.
reverse_iterator rbegin()
const_iterator end() const
bool operator!=(const Index< VDimension > &one, const Index< VDimension > &two)
SizeValueType m_InternalArray[VDimension]
static Self Filled(const IndexValueType value)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
const IndexValueType * data() const
signed long OffsetValueType
signed long IndexValueType
static Self GetBasisIndex(unsigned int dim)
::itk::IndexValueType value_type
const Self & operator+=(const SizeType &sz)
const Self operator-(const SizeType &sz) const
const value_type * const_iterator
std::reverse_iterator< iterator > reverse_iterator
constexpr unsigned int Dimension
void ExceptionThrowingBoundsCheck(size_type pos) const
const Self & operator-=(const SizeType &sz)
const Self & operator-=(const OffsetType &offset)