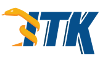 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
24 #include <type_traits>
68 template <
unsigned int VDimension = 2>
69 struct ITK_TEMPLATE_EXPORT
Size final
83 static constexpr
unsigned int Dimension = VDimension;
86 static constexpr
unsigned int
98 for (
unsigned int i = 0; i < VDimension; i++)
109 for (
unsigned int i = 0; i < VDimension; i++)
123 for (
unsigned int i = 0; i < VDimension; i++)
134 for (
unsigned int i = 0; i < VDimension; i++)
147 for (
unsigned int i = 0; i < VDimension; i++)
158 for (
unsigned int i = 0; i < VDimension; i++)
171 return m_InternalArray;
181 std::copy_n(val, VDimension, m_InternalArray);
193 m_InternalArray[element] = val;
205 return m_InternalArray[element];
213 std::fill_n(begin(), size(), value);
230 static_assert(VDimension > 0,
"Error: Only positive value sized VDimension allowed");
257 std::fill_n(begin(), size(), newValue);
269 return iterator(&m_InternalArray[0]);
281 return iterator(&m_InternalArray[VDimension]);
296 const_reverse_iterator
308 const_reverse_iterator
339 ExceptionThrowingBoundsCheck(pos);
340 return m_InternalArray[pos];
346 ExceptionThrowingBoundsCheck(pos);
347 return m_InternalArray[pos];
365 return VDimension ? *(end() - 1) : *end();
371 return VDimension ? *(end() - 1) : *end();
377 return &m_InternalArray[0];
383 return &m_InternalArray[0];
390 if (pos >= VDimension)
392 throw std::out_of_range(
"array::ExceptionThrowingBoundsCheck");
411 template <
unsigned int VDimension>
416 for (
unsigned int i = 0; i + 1 < VDimension; ++i)
418 os << obj[i] <<
", ";
422 os << obj[VDimension - 1];
430 template <
unsigned int VDimension>
437 template <
unsigned int VDimension>
441 return !(one == two);
444 template <
unsigned int VDimension>
448 return std::lexicographical_compare(one.
begin(), one.
end(), two.
begin(), two.
end());
451 template <
unsigned int VDimension>
458 template <
unsigned int VDimension>
465 template <
unsigned int VDimension>
473 template <
unsigned int VDimension>
481 template <
unsigned int VDimension>
void SetElement(unsigned long element, SizeValueType val)
reverse_iterator rbegin()
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
bool operator<=(const Index< VDimension > &one, const Index< VDimension > &two)
Represent a n-dimensional size (bounds) of a n-dimensional image.
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
void ExceptionThrowingBoundsCheck(size_type pos) const
void swap(Array< T > &a, Array< T > &b)
std::reverse_iterator< iterator > reverse_iterator
const_iterator end() const
const Self & operator+=(const Self &vec)
const_reference back() const
reference operator[](size_type pos)
const value_type & const_reference
void Fill(SizeValueType value)
const_reverse_iterator rbegin() const
const_reverse_iterator rend() const
static constexpr unsigned int GetSizeDimension()
const value_type * const_iterator
std::reverse_iterator< const_iterator > const_reverse_iterator
const Self & operator*=(const Self &vec)
::itk::SizeValueType SizeValueType
void assign(const value_type &newValue)
const Self & operator-=(const Self &vec)
bool operator>=(const Index< VDimension > &one, const Index< VDimension > &two)
::itk::SizeValueType value_type
bool operator>(const Index< VDimension > &one, const Index< VDimension > &two)
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
static Self Filled(const SizeValueType value)
const Self operator-(const Self &vec) const
std::ptrdiff_t difference_type
void SetSize(const SizeValueType val[VDimension])
const_iterator begin() const
SizeValueType GetElement(unsigned long element) const
const Self operator*(const Self &vec) const
bool operator!=(const Index< VDimension > &one, const Index< VDimension > &two)
constexpr size_type size() const
SizeValueType m_InternalArray[VDimension]
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
void swap(Size< VDimension > &one, Size< VDimension > &two)
const SizeValueType * data() const
constexpr bool empty() const
const Self operator+(const Self &vec) const
const_reference operator[](size_type pos) const
const_reference front() const
const_reference at(size_type pos) const
constexpr unsigned int Dimension
constexpr size_type max_size() const
reference at(size_type pos)
static constexpr unsigned int Dimension
unsigned long SizeValueType
const SizeValueType * GetSize() const