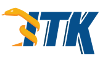 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
66 template <
unsigned int VDimension = 2>
67 struct ITK_TEMPLATE_EXPORT
Offset final
81 static constexpr
unsigned int Dimension = VDimension;
84 static constexpr
unsigned int
97 for (
unsigned int i = 0; i < VDimension; i++)
110 for (
unsigned int i = 0; i < VDimension; i++)
112 result[i] = m_InternalArray[i] + sz[i];
121 for (
unsigned int i = 0; i < VDimension; i++)
123 m_InternalArray[i] += sz[i];
133 for (
unsigned int i = 0; i < VDimension; i++)
135 m_InternalArray[i] -= sz[i];
147 for (
unsigned int i = 0; i < VDimension; i++)
158 for (
unsigned int i = 0; i < VDimension; i++)
170 for (
unsigned int i = 0; i < VDimension; i++)
184 return m_InternalArray;
194 std::copy_n(val, VDimension, m_InternalArray);
206 m_InternalArray[element] = val;
218 return m_InternalArray[element];
226 std::fill_n(begin(), size(), value);
243 static_assert(VDimension > 0,
"Error: Only positive value sized VDimension allowed");
248 template <
typename TCoordRep>
252 for (
unsigned int i = 0; i < VDimension; ++i)
254 m_InternalArray[i] = Math::Round<OffsetValueType>(point[i]);
260 template <
typename TCoordRep>
264 for (
unsigned int i = 0; i < VDimension; ++i)
266 m_InternalArray[i] = static_cast<OffsetValueType>(point[i]);
275 GetBasisOffset(
unsigned int dim);
301 std::fill_n(begin(), size(), newValue);
313 return iterator(&m_InternalArray[0]);
325 return iterator(&m_InternalArray[VDimension]);
340 const_reverse_iterator
352 const_reverse_iterator
383 ExceptionThrowingBoundsCheck(pos);
384 return m_InternalArray[pos];
390 ExceptionThrowingBoundsCheck(pos);
391 return m_InternalArray[pos];
409 return VDimension ? *(end() - 1) : *end();
415 return VDimension ? *(end() - 1) : *end();
421 return &m_InternalArray[0];
427 return &m_InternalArray[0];
434 if (pos >= VDimension)
436 throw std::out_of_range(
"array::ExceptionThrowingBoundsCheck");
442 template <
unsigned int VDimension>
453 template <
unsigned int VDimension>
458 unsigned int dimlim = VDimension - 1;
459 for (
unsigned int i = 0; i < dimlim; ++i)
461 os << ind[i] <<
", ";
465 os << ind[VDimension - 1];
473 template <
unsigned int VDimension>
480 template <
unsigned int VDimension>
484 return !(one == two);
487 template <
unsigned int VDimension>
491 return std::lexicographical_compare(one.
begin(), one.
end(), two.
begin(), two.
end());
494 template <
unsigned int VDimension>
501 template <
unsigned int VDimension>
508 template <
unsigned int VDimension>
516 template <
unsigned int VDimension>
524 template <
unsigned int VDimension>
const Self & operator-=(const Self &vec)
void CopyWithRound(const FixedArray< TCoordRep, VDimension > &point)
const OffsetValueType * GetOffset() const
void Fill(OffsetValueType value)
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
void swap(Offset< VDimension > &one, Offset< VDimension > &two)
bool operator<=(const Index< VDimension > &one, const Index< VDimension > &two)
Represent a n-dimensional size (bounds) of a n-dimensional image.
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
void SetOffset(const OffsetValueType val[VDimension])
reverse_iterator rbegin()
const_reference operator[](size_type pos) const
std::reverse_iterator< const_iterator > const_reverse_iterator
void swap(Array< T > &a, Array< T > &b)
const Self operator-(const Self &vec)
static Self GetBasisOffset(unsigned int dim)
constexpr bool empty() const
const value_type & const_reference
const_iterator end() const
const_reverse_iterator rend() const
reference operator[](size_type pos)
static constexpr unsigned int GetOffsetDimension()
const_iterator begin() const
const Self & operator-=(const Size< VDimension > &sz)
std::reverse_iterator< iterator > reverse_iterator
const OffsetValueType * data() const
const_reference back() const
constexpr size_type size() const
const Self & operator+=(const Self &vec)
const Self & operator+=(const Size< VDimension > &sz)
static constexpr unsigned int Dimension
void assign(const value_type &newValue)
bool operator>=(const Index< VDimension > &one, const Index< VDimension > &two)
constexpr size_type max_size() const
OffsetValueType m_InternalArray[VDimension]
bool operator>(const Index< VDimension > &one, const Index< VDimension > &two)
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
Simulate a standard C array with copy semantics.
void CopyWithCast(const FixedArray< TCoordRep, VDimension > &point)
Represent a n-dimensional offset between two n-dimensional indexes of n-dimensional image.
const Self operator+(const Size< VDimension > &sz) const
const Self operator+(const Self &vec) const
std::ptrdiff_t difference_type
bool operator!=(const Index< VDimension > &one, const Index< VDimension > &two)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
const_reverse_iterator rbegin() const
const_reference front() const
signed long OffsetValueType
reference at(size_type pos)
::itk::OffsetValueType OffsetValueType
OffsetValueType GetElement(unsigned long element) const
void ExceptionThrowingBoundsCheck(size_type pos) const
const_reference at(size_type pos) const
::itk::OffsetValueType value_type
constexpr unsigned int Dimension
const value_type * const_iterator
void SetElement(unsigned long element, OffsetValueType val)