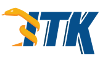 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkFixedArray_h
19 #define itkFixedArray_h
51 template <
typename TValue,
unsigned int VLength = 3>
56 static constexpr
unsigned int Length = VLength;
62 using ValueType = TValue;
73 class ConstReverseIterator;
213 explicit FixedArray(
const std::array<ValueType, VLength> & stdArray)
215 std::copy_n(stdArray.cbegin(), VLength, m_InternalArray);
219 template <
typename TFixedArrayValueType>
224 for (
auto & element : m_InternalArray)
226 element = static_cast<TValue>(*input++);
230 template <
typename TScalarValue>
233 std::copy_n(r, VLength, m_InternalArray);
237 template <
typename TFixedArrayValueType>
243 for (
auto & element : m_InternalArray)
245 element = static_cast<TValue>(*input++);
251 operator=(
const ValueType r[VLength]);
275 #if defined(__GNUC__)
276 # if (__GNUC__ == 4) && (__GNUC_MINOR__ == 9) || (__GNUC__ >= 7)
277 # pragma GCC diagnostic push
278 # pragma GCC diagnostic ignored "-Warray-bounds"
283 #if defined(__GNUC__)
284 # if (__GNUC__ == 4) && (__GNUC_MINOR__ == 9) || (__GNUC__ >= 7)
285 # pragma GCC diagnostic pop
302 m_InternalArray[index] = value;
307 return m_InternalArray[index];
315 return m_InternalArray;
321 return m_InternalArray;
327 return m_InternalArray;
333 return m_InternalArray;
349 itkLegacyMacro(ReverseIterator rBegin());
351 itkLegacyMacro(ConstReverseIterator rBegin()
const);
353 itkLegacyMacro(ReverseIterator rEnd());
355 itkLegacyMacro(ConstReverseIterator rEnd()
const);
360 return m_InternalArray;
366 return m_InternalArray;
372 return this->cbegin();
378 return m_InternalArray + VLength;
384 return m_InternalArray + VLength;
399 const_reverse_iterator
405 const_reverse_iterator
408 return this->crbegin();
417 const_reverse_iterator
423 const_reverse_iterator
426 return this->crend();
441 Fill(
const ValueType &);
455 Filled(
const ValueType &);
458 template <
typename TValue,
unsigned int VLength>
463 template <
typename TValue,
unsigned int VLength>
472 #ifndef ITK_MANUAL_INSTANTIATION
473 # include "itkFixedArray.hxx"
const_reverse_iterator rend() const
const_reference operator[](int index) const
reference operator[](long index)
FixedArray(const TScalarValue *r)
Represent a n-dimensional size (bounds) of a n-dimensional image.
ValueType[VLength] CArray
const ValueType * data() const
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
ReverseIterator operator--(int)
constexpr SizeType size() const
const ValueType & const_reference
A const reverse iterator through an array.
FixedArray(const FixedArray< TFixedArrayValueType, VLength > &r)
ConstReverseIterator operator++(int)
A reverse iterator through an array.
void swap(Array< T > &a, Array< T > &b)
reference operator[](unsigned long index)
ImageBaseType::SizeType SizeType
reference operator[](unsigned int index)
ConstReverseIterator(const ReverseIterator &rit)
const_reference GetElement(unsigned int index) const
const_reference operator[](long long index) const
FixedArray & operator=(const FixedArray< TFixedArrayValueType, VLength > &r)
ValueType * GetDataPointer()
reference operator[](int index)
reference operator[](unsigned short index)
ReverseIterator(Iterator i)
const ValueType * ConstIterator
ConstReverseIterator operator--(int)
const_iterator end() const noexcept
const_iterator cend() const noexcept
ConstReverseIterator(ConstIterator i)
const ValueType * GetDataPointer() const
bool operator!=(const ReverseIterator &rit) const
reference operator[](unsigned long long index)
ReverseIterator operator--()
ConstIterator operator->() const
ReverseIterator operator++(int)
const_reference operator[](unsigned int index) const
const_reference operator[](unsigned long index) const
const ValueType * const_pointer
ReverseIterator operator++()
reference operator[](short index)
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
const_reference operator[](unsigned short index) const
ConstReverseIterator operator--()
Simulate a standard C array with copy semantics.
bool operator!=(const FixedArray &r) const
ValueType & operator*() const
std::reverse_iterator< iterator > reverse_iterator
bool operator!=(const ConstReverseIterator &rit) const
void swap(FixedArray< TValue, VLength > &a, FixedArray< TValue, VLength > &b)
const_iterator begin() const noexcept
const_reference operator[](long index) const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
const_reverse_iterator crbegin() const
ConstReverseIterator operator++()
reverse_iterator rbegin()
Iterator operator->() const
void swap(FixedArray &other)
const ValueType & operator*() const
reference operator[](long long index)
bool operator==(const ReverseIterator &rit) const
bool operator==(const ConstReverseIterator &rit) const
const ValueType * const_iterator
constexpr unsigned int Dimension
const_reverse_iterator crend() const
const_reference operator[](short index) const
const_reverse_iterator rbegin() const
void SetElement(unsigned int index, const_reference value)
iterator begin() noexcept
std::reverse_iterator< const_iterator > const_reverse_iterator
const_reference operator[](unsigned long long index) const
const_iterator cbegin() const noexcept
FixedArray(const std::array< ValueType, VLength > &stdArray)