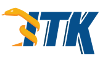 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkCovariantVector_h
19 #define itkCovariantVector_h
23 #include "vnl/vnl_vector_ref.h"
69 template <
typename T,
unsigned int NVectorDimension = 3>
86 static constexpr
unsigned int Dimension = NVectorDimension;
98 return NVectorDimension;
103 SetVnlVector(
const vnl_vector<T> &);
111 GetVnlVector()
const;
131 template <
typename TVectorValueType>
141 template <
typename TCovariantVectorValueType>
145 BaseArray::operator=(r);
152 operator=(
const ValueType r[NVectorDimension]);
155 template <
typename Tt>
159 for (
unsigned int i = 0; i < NVectorDimension; i++)
161 (*this)[i] = static_cast<ValueType>((*
this)[i] * value);
168 template <
typename Tt>
172 for (
unsigned int i = 0; i < NVectorDimension; i++)
174 (*this)[i] = static_cast<ValueType>((*
this)[i] / value);
182 operator+=(
const Self & vec);
186 operator-=(
const Self & vec);
205 ValueType
operator*(
const Self & other)
const;
217 for (
unsigned int i = 0; i < NVectorDimension; i++)
219 result[i] = static_cast<ValueType>((*
this)[i] * val);
226 template <
typename Tt>
232 for (
unsigned int i = 0; i < NVectorDimension; i++)
234 result[i] = static_cast<ValueType>((*
this)[i] / val);
247 return NVectorDimension;
256 GetSquaredNorm()
const;
260 template <
typename TCoordRepB>
264 for (
unsigned int i = 0; i < NVectorDimension; i++)
266 (*this)[i] = static_cast<T>(pa[i]);
274 template <
typename T,
unsigned int NVectorDimension>
277 return v.operator*(scalar);
282 template <
typename T,
unsigned int NVectorDimension>
286 return covariant.operator*(contravariant);
289 ITKCommon_EXPORT
void
290 CrossProduct(CovariantVector<double, 3> &,
const Vector<double, 3> &,
const Vector<double, 3> &);
292 ITKCommon_EXPORT
void
293 CrossProduct(CovariantVector<float, 3> &,
const Vector<float, 3> &,
const Vector<float, 3> &);
295 ITKCommon_EXPORT
void
296 CrossProduct(CovariantVector<int, 3>,
const Vector<int, 3> &,
const Vector<int, 3> &);
299 template <
typename T,
unsigned int NVectorDimension>
318 #ifndef ITK_MANUAL_INSTANTIATION
319 # include "itkCovariantVector.hxx"
Self operator/(const Tt &val) const
typename NumericTraits< ValueType >::RealType RealValueType
CovariantVector(const CovariantVector< TVectorValueType, NVectorDimension > &r)
void swap(Array< T > &a, Array< T > &b)
A templated class holding a n-Dimensional vector.
ConstNeighborhoodIterator< TImage > operator-(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
const Self & operator*=(const Tt &value)
static unsigned int GetCovariantVectorDimension()
static unsigned int GetNumberOfComponents()
ITKCommon_EXPORT void CrossProduct(CovariantVector< double, 3 > &, const Vector< double, 3 > &, const Vector< double, 3 > &)
Simulate a standard C array with copy semantics.
void CastFrom(const CovariantVector< TCoordRepB, NVectorDimension > &pa)
CovariantVector< T, NVectorDimension > operator*(const T &scalar, const CovariantVector< T, NVectorDimension > &v)
A templated class holding a n-Dimensional covariant vector.
const Self & operator/=(const Tt &value)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
CovariantVector(const ValueType r[Dimension])
void swap(FixedArray &other)
ConstNeighborhoodIterator< TImage > operator+(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
Self & operator=(const CovariantVector< TCovariantVectorValueType, NVectorDimension > &r)
Self operator*(const ValueType &val) const
constexpr unsigned int Dimension