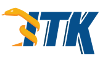 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkImageConstIteratorWithOnlyIndex_h
19 #define itkImageConstIteratorWithOnlyIndex_h
94 template <
typename TImage>
105 static constexpr
unsigned int ImageDimension = TImage::ImageDimension;
143 operator=(
const Self & it);
149 return ImageDimension;
215 return m_PositionIndex;
231 m_PositionIndex = ind;
280 #ifndef ITK_MANUAL_INSTANTIATION
281 # include "itkImageConstIteratorWithOnlyIndex.hxx"
static unsigned int GetImageDimension()
TImage::ConstPointer m_Image
IndexType m_PositionIndex
bool IsAtReverseEnd() const
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
bool operator<=(const Index< VDimension > &one, const Index< VDimension > &two)
bool operator>=(const Self &it) const
typename TImage::SizeType SizeType
typename TImage::OffsetType OffsetType
void SetIndex(const IndexType &ind)
ImageBaseType::SizeType SizeType
typename TImage::RegionType RegionType
bool operator>(const Self &it) const
typename IndexType::IndexValueType IndexValueType
const IndexType & GetIndex() const
ImageBaseType::IndexType IndexType
ImageBaseType::RegionType RegionType
bool operator==(const Self &it) const
bool operator!=(const Self &it) const
const RegionType & GetRegion() const
A base class for multi-dimensional iterators templated over image type that are designed to provide o...
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
signed long OffsetValueType
signed long IndexValueType
typename SizeType::SizeValueType SizeValueType
typename TImage::IndexType IndexType
typename OffsetType::OffsetValueType OffsetValueType
unsigned long SizeValueType