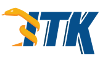 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
19 #ifndef itkLevelSetEquationContainer_h
20 #define itkLevelSetEquationContainer_h
57 template <
typename TTermContainer>
98 UpdateInternalEquationTerms();
108 InitializeParameters();
113 ComputeCFLContribution()
const;
141 : m_Iterator(it.m_Iterator)
194 return m_Iterator->first;
200 return m_Iterator->second;
216 : m_Iterator(it.m_Iterator)
273 return m_Iterator->first;
279 return m_Iterator->second;
307 #ifndef ITK_MANUAL_INSTANTIATION
308 # include "itkLevelSetEquationContainer.hxx"
311 #endif // itkLevelSetEquationContainer_h
bool operator==(const ConstIterator &it) const
bool operator!=(const Iterator &it) const
ConstIterator & operator--()
typename TermContainerType::InputImageType InputImageType
Iterator(const MapContainerIterator &it)
ConstIterator operator++(int)
bool operator==(const Iterator &it) const
typename TermContainerType::LevelSetContainerType LevelSetContainerType
LevelSetIdentifierType GetIdentifier() const
typename MapContainerType::const_iterator MapContainerConstIterator
LevelSetContainerPointer m_LevelSetContainer
bool operator!=(const Iterator &it) const
ConstIterator & operator*()
std::map< LevelSetIdentifierType, TermContainerPointer > MapContainerType
MapContainerIterator m_Iterator
typename TermContainerType::LevelSetOutputRealType LevelSetOutputRealType
bool operator==(const ConstIterator &it) const
TermContainerType * GetEquation() const
LevelSetIdentifierType GetIdentifier() const
bool operator!=(const ConstIterator &it) const
bool operator!=(const ConstIterator &it) const
typename MapContainerType::iterator MapContainerIterator
typename TermContainerType::LevelSetInputIndexType LevelSetInputIndexType
Light weight base class for most itk classes.
ConstIterator operator--(int)
bool operator==(const Iterator &it) const
MapContainerType m_Container
InputImagePointer m_Input
typename TermContainerType::InputImagePointer InputImagePointer
typename TermContainerType::Pointer TermContainerPointer
ConstIterator(const MapContainerConstIterator &it)
TTermContainer TermContainerType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
ConstIterator & operator++()
typename TermContainerType::LevelSetIdentifierType LevelSetIdentifierType
Base class for most ITK classes.
TermContainerType * GetEquation() const
MapContainerConstIterator m_Iterator
ConstIterator * operator->()
Class for holding a set of level set equations (PDEs).
ConstIterator(const Iterator &it)
Iterator(const ConstIterator &it)
typename TermContainerType::LevelSetContainerPointer LevelSetContainerPointer