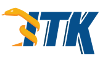 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkLevelSetFunction_h
19 #define itkLevelSetFunction_h
22 #include "vnl/vnl_matrix_fixed.h"
65 template <
typename TImageType>
84 static constexpr
unsigned int ImageDimension = Superclass::ImageDimension;
113 vnl_matrix_fixed<ScalarValueType, Self::ImageDimension, Self::ImageDimension>
m_dxy;
128 return m_ZeroVectorConstant;
133 virtual ScalarValueType
141 virtual ScalarValueType
149 virtual ScalarValueType
159 m_AdvectionWeight = a;
164 return m_AdvectionWeight;
172 m_PropagationWeight = p;
177 return m_PropagationWeight;
185 m_CurvatureWeight = c;
190 return m_CurvatureWeight;
198 m_LaplacianSmoothingWeight = c;
203 return m_LaplacianSmoothingWeight;
211 m_EpsilonMagnitude =
e;
216 return m_EpsilonMagnitude;
222 ComputeUpdate(
const NeighborhoodType & it,
224 const FloatOffsetType & = FloatOffsetType(0.0))
override;
233 ComputeGlobalTimeStep(
void * GlobalData)
const override;
257 Initialize(
const RadiusType & r);
270 virtual ScalarValueType
271 ComputeCurvatureTerm(
const NeighborhoodType &,
const FloatOffsetType &, GlobalDataStruct * gd = 0);
273 virtual ScalarValueType
274 ComputeMeanCurvature(
const NeighborhoodType &,
const FloatOffsetType &, GlobalDataStruct * gd = 0);
276 virtual ScalarValueType
277 ComputeMinimalCurvature(
const NeighborhoodType &,
const FloatOffsetType &, GlobalDataStruct * gd = 0);
279 virtual ScalarValueType
280 Compute3DMinimalCurvature(
const NeighborhoodType &,
const FloatOffsetType &, GlobalDataStruct * gd = 0);
286 m_UseMinimalCurvature = b;
292 return m_UseMinimalCurvature;
298 this->SetUseMinimalCurvature(
true);
304 this->SetUseMinimalCurvature(
false);
350 PrintSelf(std::ostream & os,
Indent indent)
const override;
357 std::slice x_slice[Self::ImageDimension];
365 bool m_UseMinimalCurvature{
false };
370 InitializeZeroVectorConstant();
392 #ifndef ITK_MANUAL_INSTANTIATION
393 # include "itkLevelSetFunction.hxx"
ScalarValueType GetPropagationWeight() const
bool GetUseMinimalCurvature() const
virtual ScalarValueType PropagationSpeed(const NeighborhoodType &, const FloatOffsetType &, GlobalDataStruct *=0) const
ScalarValueType GetEpsilonMagnitude() const
typename ConstNeighborhoodIterator< TImageType >::RadiusType RadiusType
ScalarValueType m_AdvectionWeight
void SetUseMinimalCurvature(bool b)
ScalarValueType m_GradMagSqr
virtual void SetAdvectionWeight(const ScalarValueType a)
ScalarValueType GetLaplacianSmoothingWeight() const
static void SetMaximumCurvatureTimeStep(double n)
ImageBaseType::SpacingType VectorType
ScalarValueType m_MaxPropagationChange
A templated class holding a n-Dimensional vector.
virtual void SetCurvatureWeight(const ScalarValueType c)
ScalarValueType GetCurvatureWeight() const
static double GetMaximumPropagationTimeStep()
Control indentation during Print() invocation.
virtual ScalarValueType CurvatureSpeed(const NeighborhoodType &, const FloatOffsetType &, GlobalDataStruct *=nullptr) const
void SetEpsilonMagnitude(const ScalarValueType e)
virtual VectorType AdvectionField(const NeighborhoodType &, const FloatOffsetType &, GlobalDataStruct *=0) const
ScalarValueType m_LaplacianSmoothingWeight
Light weight base class for most itk classes.
vnl_matrix_fixed< ScalarValueType, Self::ImageDimension, Self::ImageDimension > m_dxy
ScalarValueType m_MaxCurvatureChange
virtual ScalarValueType LaplacianSmoothingSpeed(const NeighborhoodType &, const FloatOffsetType &, GlobalDataStruct *=0) const
void SetLaplacianSmoothingWeight(const ScalarValueType c)
ScalarValueType m_MaxAdvectionChange
Simulate a standard C array with copy semantics.
Define additional traits for native types such as int or float.
virtual void SetPropagationWeight(const ScalarValueType p)
PixelType ScalarValueType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
static VectorType m_ZeroVectorConstant
Const version of NeighborhoodIterator, defining iteration of a local N-dimensional neighborhood of pi...
static void SetMaximumPropagationTimeStep(double n)
signed long OffsetValueType
void UseMinimalCurvatureOn()
ScalarValueType m_CurvatureWeight
ScalarValueType m_EpsilonMagnitude
ScalarValueType GetAdvectionWeight() const
static constexpr double e
The LevelSetFunction class is a generic function object which can be used to create a level set metho...
void * GetGlobalDataPointer() const override
typename Superclass::PixelType PixelType
void UseMinimalCurvatureOff()
void ReleaseGlobalDataPointer(void *GlobalData) const override
static double GetMaximumCurvatureTimeStep()
ScalarValueType m_PropagationWeight