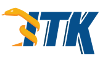 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
25 #include "vnl/vnl_vector_ref.h"
52 template <
typename TCoordRep,
unsigned int NPo
intDimension = 3>
69 static constexpr
unsigned int PointDimension = NPointDimension;
80 return NPointDimension;
91 operator=(
const Point &) =
default;
93 operator=(
Point &&) =
default;
97 template <
typename TPo
intValueType>
103 template <
typename TPo
intValueType>
104 Point(
const TPointValueType r[NPointDimension])
112 template <
typename TPo
intValueType>
122 explicit Point(
const std::array<ValueType, NPointDimension> & stdArray)
128 operator=(
const ValueType r[NPointDimension]);
136 for (
unsigned int i = 0; i < NPointDimension && same; ++i)
149 for (
unsigned int i = 0; i < NPointDimension && same; ++i)
178 GetVectorFromOrigin()
const;
181 vnl_vector_ref<TCoordRep>
185 vnl_vector<TCoordRep>
186 GetVnlVector()
const;
200 SetToMidPoint(
const Self &,
const Self &);
229 SetToBarycentricCombination(
const Self & A,
const Self & B,
double alpha);
248 SetToBarycentricCombination(
const Self & A,
const Self & B,
const Self & C,
double weightForA,
double weightForB);
264 SetToBarycentricCombination(
const Self * P,
const double * weights,
unsigned int N);
269 template <
typename TCoordRepB>
273 for (
unsigned int i = 0; i < NPointDimension; i++)
275 (*this)[i] = static_cast<TCoordRep>(pa[i]);
284 template <
typename TCoordRepB>
290 for (
unsigned int i = 0; i < NPointDimension; i++)
292 const auto component = static_cast<RealType>(pa[i]);
293 const RealType difference = static_cast<RealType>((*
this)[i]) - component;
294 sum += difference * difference;
302 template <
typename TCoordRepB>
306 const double distance = std::sqrt(static_cast<double>(this->SquaredEuclideanDistanceTo(pa)));
308 return static_cast<RealType>(distance);
312 template <
typename T,
unsigned int NPo
intDimension>
314 operator<<(std::ostream & os,
const Point<T, NPointDimension> & vct);
316 template <
typename T,
unsigned int NPo
intDimension>
318 operator>>(std::istream & is, Point<T, NPointDimension> & vct);
346 template <
typename TPo
intContainer,
typename TWeightContainer>
365 template <
typename TCoordRep,
unsigned int NPo
intDimension>
374 #ifndef ITK_MANUAL_INSTANTIATION
375 # include "itkPoint.hxx"
Computes the barycentric combination of an array of N points.
TWeightContainer WeightContainerType
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
RealType SquaredEuclideanDistanceTo(const Point< TCoordRepB, NPointDimension > &pa) const
Point(const std::array< ValueType, NPointDimension > &stdArray)
ImageBaseType::SpacingType VectorType
bool ExactlyEquals(const TInput1 &x1, const TInput2 &x2)
Return the result of an exact comparison between two scalar values of potentially different types.
void swap(Array< T > &a, Array< T > &b)
A templated class holding a n-Dimensional vector.
ConstNeighborhoodIterator< TImage > operator-(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
Point(const TPointValueType &v)
static unsigned int GetPointDimension()
const ValueType * ConstIterator
Point(const TPointValueType r[NPointDimension])
typename NumericTraits< ValueType >::RealType RealType
std::istream & operator>>(std::istream &is, Point< T, NPointDimension > &vct)
typename PointContainerType::Element PointType
Point(const Point< TPointValueType, NPointDimension > &r)
Simulate a standard C array with copy semantics.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename PointContainerType::Pointer PointContainerPointer
RealType EuclideanDistanceTo(const Point< TCoordRepB, NPointDimension > &pa) const
void swap(FixedArray &other)
ConstNeighborhoodIterator< TImage > operator+(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
A templated class holding a geometric point in n-Dimensional space.
void CastFrom(const Point< TCoordRepB, NPointDimension > &pa)
Point(const ValueType &v)
TPointContainer PointContainerType
bool operator==(const Self &pt) const
bool operator!=(const Self &pt) const
Point(const ValueType r[NPointDimension])