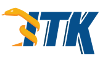 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
61 template <
typename TMeasurementVector>
65 ITK_DISALLOW_COPY_AND_MOVE(
Sample);
136 itkExceptionMacro(
"Attempting to change the measurement \
137 vector size of a non-empty Sample");
153 if (defaultLength != s)
155 itkExceptionMacro(
"Attempting to change the measurement \
156 vector size of a non-resizable vector type");
170 const auto * thatConst = dynamic_cast<const Self *>(thatObject);
IdentifierType InstanceIdentifier
virtual AbsoluteFrequencyType GetFrequency(InstanceIdentifier id) const =0
TMeasurementVector MeasurementVectorType
void Graft(const DataObject *thatObject) override
virtual InstanceIdentifier Size() const =0
virtual void Graft(const DataObject *)
NumericTraits< AbsoluteFrequencyType >::AccumulateType TotalAbsoluteFrequencyType
Control indentation during Print() invocation.
virtual TotalAbsoluteFrequencyType GetTotalFrequency() const =0
typename MeasurementVectorTraits::InstanceIdentifier InstanceIdentifier
typename MeasurementVectorTraitsTypes< MeasurementVectorType >::ValueType MeasurementType
unsigned int MeasurementVectorSizeType
InstanceIdentifier AbsoluteFrequencyType
virtual void SetMeasurementVectorSize(MeasurementVectorSizeType s)
~Sample() override=default
void PrintSelf(std::ostream &os, Indent indent) const override
MeasurementVectorTraits::AbsoluteFrequencyType AbsoluteFrequencyType
MeasurementVectorSizeType m_MeasurementVectorSize
Simulate a standard C array with copy semantics.
static bool IsResizable(const TVectorType &)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
static unsigned int GetLength()
typename TMeasurementVector::ValueType ValueType
A collection of measurements for statistical analysis.
Base class for most ITK classes.
virtual const MeasurementVectorType & GetMeasurementVector(InstanceIdentifier id) const =0
void PrintSelf(std::ostream &os, Indent indent) const override
virtual void Modified() const
Base class for all data objects in ITK.