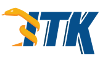 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkScalarImageToRunLengthMatrixFilter_h
19 #define itkScalarImageToRunLengthMatrixFilter_h
106 template <
typename TImageType,
typename THistogramFrequencyContainer = DenseFrequencyContainer2>
143 static constexpr
unsigned int ImageDimension = TImageType::ImageDimension;
146 static constexpr
unsigned int DefaultBinsPerAxis = 256;
177 itkSetMacro(NumberOfBinsPerAxis,
unsigned int);
180 itkGetConstMacro(NumberOfBinsPerAxis,
unsigned int);
205 itkGetConstMacro(MinDistance,
RealType);
210 itkGetConstMacro(MaxDistance,
RealType);
213 using Superclass::SetInput;
227 GetMaskImage()
const;
237 itkSetMacro(InsidePixelValue,
PixelType);
238 itkGetConstMacro(InsidePixelValue,
PixelType);
245 PrintSelf(std::ostream & os,
Indent indent)
const override;
251 using Superclass::MakeOutput;
257 GenerateData()
override;
267 NormalizeOffsetDirection(
OffsetType & offset);
284 #ifndef ITK_MANUAL_INSTANTIATION
285 # include "itkScalarImageToRunLengthMatrixFilter.hxx"
This class computes a run length matrix (histogram) from a given image and a mask image if provided....
typename NumericTraits< PixelType >::RealType RealType
unsigned int m_NumberOfBinsPerAxis
MeasurementVectorType m_LowerBound
ImageBaseType::PointType PointType
ImageBaseType::SizeType SizeType
typename OffsetVector::Pointer OffsetVectorPointer
Control indentation during Print() invocation.
DataObjectPointerArray::size_type DataObjectPointerArraySizeType
OffsetVectorPointer m_Offsets
typename ImageType::PixelType PixelType
typename ImageType::IndexType IndexType
typename ImageType::PointType PointType
ImageBaseType::IndexType IndexType
typename HistogramType::MeasurementVectorType MeasurementVectorType
typename Superclass::MeasurementVectorType MeasurementVectorType
This class stores measurement vectors in the context of n-dimensional histogram.
Light weight base class for most itk classes.
ImageBaseType::RegionType RegionType
typename ImageType::Pointer ImagePointer
typename HistogramType::Pointer HistogramPointer
typename HistogramType::ConstPointer HistogramConstPointer
MeasurementVectorType m_UpperBound
Define additional traits for native types such as int or float.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
class ITK_FORWARD_EXPORT ProcessObject
typename ImageType::RegionType RegionType
typename ImageType::OffsetType OffsetType
typename NumericTraits< PixelType >::RealType MeasurementType
SmartPointer< Self > Pointer
Define a front-end to the STL "vector" container that conforms to the IndexedContainerInterface.
PixelType m_InsidePixelValue
typename ImageType::ConstPointer ImageConstPointer
typename ImageType::SizeType RadiusType