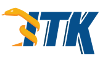 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkHistogram_h
19 #define itkHistogram_h
76 template <
typename TMeasurement =
float,
typename TFrequencyContainer = DenseFrequencyContainer2>
161 itkGetConstMacro(ClipBinsAtEnds,
bool);
165 itkSetMacro(ClipBinsAtEnds,
bool);
170 IsIndexOutOfBounds(
const IndexType & index)
const;
176 GetInstanceIdentifier(
const IndexType & index)
const;
180 Size()
const override;
188 GetSize(
unsigned int dimension)
const;
209 GetBinMinFromValue(
unsigned int dimension,
float value)
const;
214 GetBinMaxFromValue(
unsigned int dimension,
float value)
const;
218 GetDimensionMins(
unsigned int dimension)
const;
222 GetDimensionMaxs(
unsigned int dimension)
const;
234 GetHistogramMinFromIndex(
const IndexType & index)
const;
238 GetHistogramMaxFromIndex(
const IndexType & index)
const;
246 GetFrequency(
const IndexType & index)
const;
297 GetMeasurementVector(
const IndexType & index)
const;
306 GetTotalFrequency()
const override;
328 Quantile(
unsigned int dimension,
double p)
const;
333 Mean(
unsigned int dimension)
const;
341 PrintSelf(std::ostream & os,
Indent indent)
const override;
357 m_Histogram = histogram;
377 return m_Histogram->GetFrequency(m_Id);
389 return m_Histogram->GetMeasurementVector(m_Id);
395 return m_Histogram->GetIndex(m_Id);
408 return (m_Id != it.
m_Id);
414 return (m_Id == it.
m_Id);
420 , m_Histogram(histogram)
464 this->ConstIterator::operator=(it);
471 auto * histogram = const_cast<Self *>(this->m_Histogram);
473 return histogram->SetFrequency(this->m_Id, value);
488 return Iterator(m_OffsetTable[this->GetMeasurementVectorSize()],
this);
502 return ConstIterator(m_OffsetTable[this->GetMeasurementVectorSize()],
this);
516 unsigned int m_NumberOfInstances{ 0 };
525 std::vector<std::vector<MeasurementType>>
m_Min;
528 std::vector<std::vector<MeasurementType>>
m_Max;
533 bool m_ClipBinsAtEnds{
true };
538 #ifndef ITK_MANUAL_INSTANTIATION
539 # include "itkHistogram.hxx"
class that walks through the elements of the histogram.
Iterator & operator=(const Iterator &it)
InstanceIdentifier GetInstanceIdentifier() const
typename FrequencyContainerType::Pointer FrequencyContainerPointer
Iterator(const Iterator &it)
ConstIterator(const Self *histogram)
Represent a n-dimensional size (bounds) of a n-dimensional image.
void Initialize() override
TMeasurementVector MeasurementVectorType
bool operator!=(const ConstIterator &it) const
std::vector< MeasurementType > BinMinVectorType
ConstIterator & operator++()
ConstIterator Begin() const
Control indentation during Print() invocation.
OffsetTableType m_OffsetTable
std::vector< BinMaxVectorType > BinMaxContainerType
typename Superclass::MeasurementVectorType MeasurementVectorType
This class stores measurement vectors in the context of n-dimensional histogram.
bool operator==(const ConstIterator &it) const
std::vector< BinMinVectorType > BinMinContainerType
typename FrequencyContainerType::TotalAbsoluteFrequencyType TotalAbsoluteFrequencyType
MeasurementVectorType m_TempMeasurementVector
typename FrequencyContainerType::RelativeFrequencyType RelativeFrequencyType
typename SizeType::ValueType SizeValueType
typename FrequencyContainerType::AbsoluteFrequencyType AbsoluteFrequencyType
Iterator(Self *histogram)
MeasurementVectorType ValueType
FrequencyContainerPointer m_FrequencyContainer
ConstIterator & operator=(const ConstIterator &it)
std::vector< MeasurementType > BinMaxVectorType
typename FrequencyContainerType::TotalRelativeFrequencyType TotalRelativeFrequencyType
std::vector< std::vector< MeasurementType > > m_Min
std::vector< InstanceIdentifier > OffsetTableType
typename Superclass::MeasurementVectorSizeType MeasurementVectorSizeType
::itk::IndexValueType ValueType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
AbsoluteFrequencyType GetFrequency() const
std::vector< std::vector< MeasurementType > > m_Max
typename IndexType::ValueType IndexValueType
Array class with size defined at construction time.
TFrequencyContainer FrequencyContainerType
A collection of measurements for statistical analysis.
Base class for most ITK classes.
const MeasurementVectorType & GetMeasurementVector() const
Iterator(InstanceIdentifier id, Self *histogram)
const IndexType & GetIndex() const
TMeasurement MeasurementType
class that walks through the elements of the histogram.
ConstIterator End() const
ConstIterator(InstanceIdentifier id, const Self *histogram)
typename Superclass::InstanceIdentifier InstanceIdentifier
ConstIterator(const ConstIterator &it)
bool SetFrequency(const AbsoluteFrequencyType value)
Base class for all data objects in ITK.