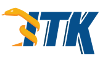 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkShapePriorSegmentationLevelSetFunction_h
19 #define itkShapePriorSegmentationLevelSetFunction_h
54 template <
typename TImageType,
typename TFeatureImageType = TImageType>
88 static constexpr
unsigned int ImageDimension = Superclass::ImageDimension;
98 m_ShapePriorWeight = p;
103 return m_ShapePriorWeight;
112 m_ShapeFunction = ptr;
114 const ShapeFunctionType *
117 return m_ShapeFunction;
123 ComputeUpdate(
const NeighborhoodType & neighborhood,
125 const FloatOffsetType & = FloatOffsetType(0.0))
override;
129 ComputeGlobalTimeStep(
void * gd)
const override;
164 PrintSelf(std::ostream & os,
Indent indent)
const override;
172 #ifndef ITK_MANUAL_INSTANTIATION
173 # include "itkShapePriorSegmentationLevelSetFunction.hxx"
ScalarValueType GetShapePriorWeight() const
typename ConstNeighborhoodIterator< TImageType >::RadiusType RadiusType
ScalarValueType m_ShapePriorWeight
ScalarValueType m_MaxShapePriorChange
typename ShapeFunctionType::ConstPointer ShapeFunctionPointer
void SetShapeFunction(const ShapeFunctionType *ptr)
A templated class holding a n-Dimensional vector.
This function is used in ShapePriorSegmentationLevelSetFilter to segment structures in an image based...
typename Superclass::GlobalDataStruct GlobalDataStruct
Control indentation during Print() invocation.
const ShapeFunctionType * GetShapeFunction() const
TFeatureImageType FeatureImageType
ImageBaseType::IndexType IndexType
typename Superclass::GlobalDataStruct GlobalDataStruct
Light weight base class for most itk classes.
typename FeatureImageType::PixelType FeatureScalarType
Base class for functions which evaluates the signed distance from a shape.
void * GetGlobalDataPointer() const override
void ReleaseGlobalDataPointer(void *GlobalData) const override
typename Superclass::ScalarValueType ScalarValueType
void SetShapePriorWeight(const ScalarValueType p)
typename ImageType::PixelType PixelType
ShapeFunctionPointer m_ShapeFunction
PixelType ScalarValueType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Const version of NeighborhoodIterator, defining iteration of a local N-dimensional neighborhood of pi...
typename ImageType::IndexType IndexType
Templated n-dimensional image class.
typename InterpolatorType::ContinuousIndexType ContinuousIndexType