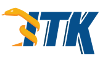 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
19 #ifndef itkShapedImageNeighborhoodRange_h
20 #define itkShapedImageNeighborhoodRange_h
27 #include <type_traits>
90 template <
typename TImage,
91 typename TImageNeighborhoodPixelAccessPolicy = ZeroFluxNeumannImageNeighborhoodPixelAccessPolicy<TImage>>
108 template <
typename T>
110 Test(
typename T::PixelAccessParameterType *);
112 template <
typename T>
119 !std::is_same<decltype(Test<TImageNeighborhoodPixelAccessPolicy>(
nullptr)),
120 decltype(Test<TImageNeighborhoodPixelAccessPolicy>())>::value;
124 template <
typename TPolicy,
bool VPolicyHasPixelAccessParameterType = CheckPolicy::HasPixelAccessParameterType>
127 using Type =
typename TPolicy::PixelAccessParameterType;
131 template <
typename TPolicy>
161 template <
bool VIsConst,
typename TDummy =
void>
167 template <
typename TDummy>
189 const TImageNeighborhoodPixelAccessPolicy & pixelAccessPolicy) ITK_NOEXCEPT
191 , m_PixelAccessPolicy{ pixelAccessPolicy }
197 , m_PixelAccessPolicy{ pixelProxy.m_PixelAccessPolicy }
207 template <
typename TDummy>
231 const TImageNeighborhoodPixelAccessPolicy & pixelAccessPolicy) ITK_NOEXCEPT
233 , m_PixelAccessPolicy{ pixelAccessPolicy }
262 const auto lhsPixelValue = lhs.m_PixelAccessPolicy.GetPixelValue(lhs.m_ImageBufferPointer);
263 const auto rhsPixelValue = rhs.m_PixelAccessPolicy.GetPixelValue(rhs.m_ImageBufferPointer);
266 lhs.m_PixelAccessPolicy.SetPixelValue(lhs.m_ImageBufferPointer, rhsPixelValue);
267 rhs.m_PixelAccessPolicy.SetPixelValue(rhs.m_ImageBufferPointer, lhsPixelValue);
284 template <
bool VIsConst>
302 typename std::conditional<IsImageTypeConst, const InternalPixelType, InternalPixelType>::type;
305 using QualifiedPixelType =
typename std::conditional<IsImageTypeConst, const PixelType, PixelType>::type;
353 return TImageNeighborhoodPixelAccessPolicy{
358 template <
typename TPixelAccessParameter>
359 TImageNeighborhoodPixelAccessPolicy
362 static_assert(std::is_same<TPixelAccessParameter, OptionalPixelAccessParameterType>::value,
363 "This helper function should only be used for OptionalPixelAccessParameterType!");
364 static_assert(!std::is_same<TPixelAccessParameter, EmptyPixelAccessParameter>::value,
365 "EmptyPixelAccessParameter indicates that there is no pixel access parameter specified!");
366 return TImageNeighborhoodPixelAccessPolicy{
469 assert(lhs.m_ImageBufferPointer == rhs.m_ImageBufferPointer);
470 assert(lhs.m_ImageSize == rhs.m_ImageSize);
471 assert(lhs.m_OffsetTable == rhs.m_OffsetTable);
474 return lhs.m_CurrentOffset == rhs.m_CurrentOffset;
483 return !(lhs == rhs);
491 assert(lhs.m_ImageBufferPointer == rhs.m_ImageBufferPointer);
492 assert(lhs.m_ImageSize == rhs.m_ImageSize);
493 assert(lhs.m_OffsetTable == rhs.m_OffsetTable);
496 return lhs.m_CurrentOffset < rhs.m_CurrentOffset;
549 assert(lhs.m_ImageBufferPointer == rhs.m_ImageBufferPointer);
550 assert(lhs.m_ImageSize == rhs.m_ImageSize);
551 assert(lhs.m_OffsetTable == rhs.m_OffsetTable);
554 return lhs.m_CurrentOffset - rhs.m_CurrentOffset;
594 typename std::conditional<IsImageTypeConst, const InternalPixelType, InternalPixelType>::type;
606 :
m_Index(imageRegion.GetIndex())
607 ,
m_Size(imageRegion.GetSize())
617 index1[i] -= index2[i];
670 const std::size_t numberOfNeigborhoodPixels,
685 assert(offsetTable !=
nullptr);
703 template <
typename TContainerOfOffsets>
706 const TContainerOfOffsets & shapeOffsets,
712 optionalPixelAccessParameter }
742 return this->
begin();
804 assert(n < this->
size());
805 assert(n <= static_cast<std::size_t>(std::numeric_limits<std::ptrdiff_t>::max()));
808 return this->
begin()[static_cast<std::ptrdiff_t>(n)];
QualifiedIterator(QualifiedInternalPixelType *const imageBufferPointer, const ImageSizeType &imageSize, const OffsetType &offsetTable, const NeighborhoodAccessorFunctorType &neighborhoodAccessor, const OptionalPixelAccessParameterType optionalPixelAccessParameter, const IndexType &relativeLocation, const OffsetType *const offset) noexcept
const_iterator cend() const noexcept
RegionData m_BufferedRegionData
const_reverse_iterator crbegin() const noexcept
typename TImage::IndexValueType IndexValueType
PixelProxy(InternalPixelType *const imageBufferPointer, const TImageNeighborhoodPixelAccessPolicy &pixelAccessPolicy) noexcept
typename TImage::SizeType ImageSizeType
static constexpr bool IsImageTypeConst
QualifiedIterator operator++(int) noexcept
const_reverse_iterator crend() const noexcept
QualifiedPixelType * pointer
std::vcl_size_t size() const noexcept
PixelProxy & operator=(const PixelType &pixelValue) noexcept
QualifiedIterator operator--(int) noexcept
iterator begin() const noexcept
IndexType m_RelativeLocation
friend bool operator==(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
typename TImage::RegionType ImageRegionType
QualifiedIterator< true > const_iterator
friend QualifiedIterator operator+(const difference_type n, QualifiedIterator it) noexcept
NeighborhoodAccessorFunctorType m_NeighborhoodAccessor
typename TImage::PixelType PixelType
friend bool operator!=(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
ImageBaseType::SizeType SizeType
typename std::conditional< IsImageTypeConst, const PixelType, PixelType >::type QualifiedPixelType
void SetLocation(const IndexType &location) noexcept
std::vcl_size_t m_NumberOfNeighborhoodPixels
InternalPixelType *const m_ImageBufferPointer
std::reverse_iterator< iterator > reverse_iterator
PixelProxy(const PixelProxy< false > &pixelProxy) noexcept
QualifiedIterator()=default
const_iterator cbegin() const noexcept
static int Test(typename T::PixelAccessParameterType *)
friend difference_type operator-(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
OptionalPixelAccessParameterType m_OptionalPixelAccessParameter
friend bool operator<(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
friend QualifiedIterator operator-(QualifiedIterator it, const difference_type n) noexcept
QualifiedIterator< false >::reference operator[](const std::vcl_size_t n) const noexcept
friend void swap(PixelProxy lhs, PixelProxy rhs) noexcept
QualifiedIterator & operator=(const QualifiedIterator &) noexcept=default
ImageBaseType::IndexType IndexType
const TImageNeighborhoodPixelAccessPolicy m_PixelAccessPolicy
static constexpr bool HasPixelAccessParameterType
IndexType m_RelativeLocation
const TImageNeighborhoodPixelAccessPolicy m_PixelAccessPolicy
OptionalPixelAccessParameterType m_OptionalPixelAccessParameter
reference operator*() const noexcept
PixelProxy & operator=(const PixelProxy &pixelProxy) noexcept
const InternalPixelType *const m_ImageBufferPointer
std::ptrdiff_t difference_type
const OffsetType * m_ShapeOffsets
friend QualifiedIterator & operator+=(QualifiedIterator &it, const difference_type n) noexcept
friend bool operator>(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
typename TImage::NeighborhoodAccessorFunctorType NeighborhoodAccessorFunctorType
typename TImage::SizeValueType ImageSizeValueType
QualifiedInternalPixelType * m_ImageBufferPointer
static constexpr bool IsImageTypeConst
ImageBaseType::RegionType RegionType
QualifiedIterator(const QualifiedIterator< false > &arg) noexcept
QualifiedIterator & operator--() noexcept
RegionData() noexcept=default
friend bool operator<=(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
typename std::conditional< IsImageTypeConst, const InternalPixelType, InternalPixelType >::type QualifiedInternalPixelType
typename std::conditional< IsImageTypeConst, const InternalPixelType, InternalPixelType >::type QualifiedInternalPixelType
reverse_iterator rend() const noexcept
QualifiedIterator & operator++() noexcept
QualifiedInternalPixelType * m_ImageBufferPointer
typename std::conditional< VIsConst, const ImageType, ImageType >::type QualifiedImageType
std::reverse_iterator< const_iterator > const_reverse_iterator
ImageSizeType m_ImageSize
void SubtractIndex(IndexType &index1, const IndexType &index2)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
friend bool operator>=(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
signed long OffsetValueType
QualifiedIterator< IsImageTypeConst > iterator
friend QualifiedIterator & operator-=(QualifiedIterator &it, const difference_type n) noexcept
ShapedImageNeighborhoodRange(ImageType &image, const IndexType &location, const TContainerOfOffsets &shapeOffsets, const OptionalPixelAccessParameterType optionalPixelAccessParameter={})
signed long IndexValueType
bool empty() const noexcept
ShapedImageNeighborhoodRange()=default
typename OptionalPixelAccessParameter< TImageNeighborhoodPixelAccessPolicy >::Type OptionalPixelAccessParameterType
TImageNeighborhoodPixelAccessPolicy CreatePixelAccessPolicy(EmptyPixelAccessParameter) const
TImageNeighborhoodPixelAccessPolicy CreatePixelAccessPolicy(const TPixelAccessParameter pixelAccessParameter) const
std::random_access_iterator_tag iterator_category
NeighborhoodAccessorFunctorType m_NeighborhoodAccessor
typename TImage::ImageDimensionType ImageDimensionType
typename TImage::IndexType IndexType
reverse_iterator rbegin() const noexcept
iterator end() const noexcept
reference operator[](const difference_type n) const noexcept
PixelProxy(const InternalPixelType *const imageBufferPointer, const TImageNeighborhoodPixelAccessPolicy &pixelAccessPolicy) noexcept
const OffsetType * m_CurrentOffset
static constexpr ImageDimensionType ImageDimension
friend QualifiedIterator operator+(QualifiedIterator it, const difference_type n) noexcept
typename TImageNeighborhoodPixelAccessPolicy ::PixelAccessParameterType Type
typename TImage::InternalPixelType InternalPixelType
unsigned long SizeValueType
ShapedImageNeighborhoodRange(ImageType &image, const IndexType &location, const OffsetType *const shapeOffsets, const std::vcl_size_t numberOfNeigborhoodPixels, const OptionalPixelAccessParameterType optionalPixelAccessParameter={})