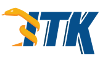 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkSparseFieldLayer_h
19 #define itkSparseFieldLayer_h
35 template <
typename TNodeType>
39 const TNodeType &
operator*()
const {
return *m_Pointer; }
41 const TNodeType *
operator->()
const {
return m_Pointer; }
78 m_Pointer = m_Pointer->Next;
85 m_Pointer = m_Pointer->Previous;
103 template <
typename TNodeType>
124 return this->m_Pointer;
130 this->m_Pointer = this->m_Pointer->Next;
137 this->m_Pointer = this->m_Pointer->Previous;
144 this->m_Pointer = const_cast<TNodeType *>(sc.
GetPointer());
171 template <
typename TNodeType>
216 return m_HeadNode->Next;
223 return m_HeadNode->Next;
230 m_HeadNode->Next = m_HeadNode->Next->Next;
231 m_HeadNode->Next->Previous = m_HeadNode;
239 n->Next = m_HeadNode->Next;
240 n->Previous = m_HeadNode;
241 m_HeadNode->Next->Previous = n;
242 m_HeadNode->Next = n;
250 n->Previous->Next = n->Next;
251 n->Next->Previous = n->Previous;
289 if (m_HeadNode->Next == m_HeadNode)
308 SplitRegions(
int num)
const;
314 PrintSelf(std::ostream & os,
Indent indent)
const override;
324 #ifndef ITK_MANUAL_INSTANTIATION
325 # include "itkSparseFieldLayer.hxx"
SparseFieldLayerIterator()
std::vector< RegionType > RegionListType
ConstSparseFieldLayerIterator & operator++()
Represent a n-dimensional size (bounds) of a n-dimensional image.
SparseFieldLayerIterator(TNodeType *p)
SparseFieldLayerIterator & operator++()
ConstSparseFieldLayerIterator(TNodeType *p)
bool operator!=(const ConstSparseFieldLayerIterator o) const
const TNodeType & operator*() const
Control indentation during Print() invocation.
Used to iterate through an itkSparseFieldLayer.
SparseFieldLayerIterator & operator=(Superclass &sc)
const TNodeType * GetPointer() const
A very simple linked list that is used to manage nodes in a layer of a sparse field level-set solver.
Light weight base class for most itk classes.
const NodeType * Front() const
bool operator==(const ConstSparseFieldLayerIterator o) const
The non-const version of the ConstSparseFieldLayerIterator.
const TNodeType * operator->() const
ConstSparseFieldLayerIterator()
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
ConstIterator Begin() const
Base class for most ITK classes.
ConstSparseFieldLayerIterator & operator--()
ConstIterator End() const
void PushFront(NodeType *n)
SparseFieldLayerIterator & operator--()