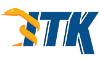 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkAdaptImageFilter_h
19 #define itkAdaptImageFilter_h
42 template <
typename TInput,
typename TAccessor>
120 template <
typename TInputImage,
typename TOutputImage,
typename TAccessor>
124 Functor::AccessorFunctor<typename TInputImage::PixelType, TAccessor>>
typename TAccessor::ExternalType OutputType
Implements pixel-wise generic operation on one image.
~AdaptImageFilter() override=default
bool operator==(const Self &other) const
Convert an image to another pixel type using the specified data accessor.
OutputType operator()(const TInput &A) const
void SetFunctor(const FunctorType &functor)
Convert an accessor to a functor so that it can be used in a UnaryFunctorImageFilter.
Base class for all process objects that output image data.
ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(Self)
void SetAccessor(AccessorType &accessor)
void SetAccessor(AccessorType &accessor)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
AdaptImageFilter()=default
FunctorType & GetFunctor()
AccessorType & GetAccessor()
virtual void Modified() const
AccessorType & GetAccessor()