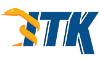 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkUnaryFunctorImageFilter_h
19 #define itkUnaryFunctorImageFilter_h
49 template <
typename TInputImage,
typename TOutputImage,
typename TFunction>
105 if (m_Functor != functor)
126 GenerateOutputInformation()
override;
139 DynamicThreadedGenerateData(
const OutputImageRegionType & outputRegionForThread)
override;
146 #ifndef ITK_MANUAL_INSTANTIATION
147 # include "itkUnaryFunctorImageFilter.hxx"
SmartPointer< Self > Pointer
SmartPointer< const Self > ConstPointer
typename OutputImageType::Pointer OutputImagePointer
Implements pixel-wise generic operation on one image.
const FunctorType & GetFunctor() const
Base class for filters that take an image as input and overwrite that image as the output.
void SetFunctor(const FunctorType &functor)
typename InputImageType::PixelType InputImagePixelType
Light weight base class for most itk classes.
typename InputImageType::Pointer InputImagePointer
ImageBaseType::RegionType RegionType
Functor::Add2< typename TInputImage1::PixelType, typename TInputImage2::PixelType, typename TOutputImage::PixelType > FunctorType
typename OutputImageType::RegionType OutputImageRegionType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename OutputImageType::PixelType OutputImagePixelType
FunctorType & GetFunctor()
typename InputImageType::RegionType InputImageRegionType