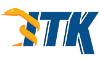 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkBinaryCrossStructuringElement_h
19 #define itkBinaryCrossStructuringElement_h
51 template <
typename TPixel,
unsigned int VDimension = 2,
typename TAllocator = NeighborhoodAllocator<TPixel>>
63 static constexpr
unsigned int NeighborhoodDimension = VDimension;
71 using Iterator =
typename AllocatorType::iterator;
94 Self::SetRadius(radius);
95 Self::CreateStructuringElement();
111 Superclass::operator=(other);
118 CreateStructuringElement();
125 #ifndef ITK_MANUAL_INSTANTIATION
126 # include "itkBinaryCrossStructuringElement.hxx"
Represent a n-dimensional size (bounds) of a n-dimensional image.
A flexible iterator for itk containers(i.e. itk::Neighborhood) that support pixel access through oper...
BinaryCrossStructuringElement()
A light-weight container object for storing an N-dimensional neighborhood of values.
ImageBaseType::SizeType SizeType
typename OffsetType::OffsetValueType OffsetValueType
void Fill(SizeValueType value)
BinaryCrossStructuringElement(const Self &other)
A Neighborhood that represents a cross structuring element with binary elements.
typename AllocatorType::iterator Iterator
typename AllocatorType::const_iterator ConstIterator
Represent a n-dimensional offset between two n-dimensional indexes of n-dimensional image.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Self & operator=(const Self &other)
unsigned long SizeValueType