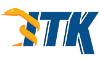 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
26 #include <type_traits>
68 template <
unsigned int VDimension = 2>
69 struct ITK_TEMPLATE_EXPORT
Size final
83 static constexpr
unsigned int Dimension = VDimension;
86 static constexpr
unsigned int
98 for (
unsigned int i = 0; i < VDimension; ++i)
109 for (
unsigned int i = 0; i < VDimension; ++i)
123 for (
unsigned int i = 0; i < VDimension; ++i)
134 for (
unsigned int i = 0; i < VDimension; ++i)
148 for (
unsigned int i = 0; i < VDimension; ++i)
159 for (
unsigned int i = 0; i < VDimension; ++i)
172 return m_InternalArray;
182 std::copy_n(val, VDimension, m_InternalArray);
194 m_InternalArray[element] = val;
206 return m_InternalArray[element];
214 std::fill_n(begin(), size(), value);
244 static_assert(VDimension > 0,
"Error: Only positive value sized VDimension allowed");
271 std::fill_n(begin(), size(), newValue);
277 std::swap(m_InternalArray, other.m_InternalArray);
280 constexpr const_iterator
283 return &m_InternalArray[0];
289 return &m_InternalArray[0];
292 constexpr const_iterator
295 return &m_InternalArray[0];
298 constexpr const_iterator
301 return &m_InternalArray[VDimension];
307 return &m_InternalArray[VDimension];
310 constexpr const_iterator
313 return &m_InternalArray[VDimension];
322 const_reverse_iterator
334 const_reverse_iterator
361 return m_InternalArray[pos];
364 constexpr const_reference
367 return m_InternalArray[pos];
373 ExceptionThrowingBoundsCheck(pos);
374 return m_InternalArray[pos];
380 ExceptionThrowingBoundsCheck(pos);
381 return m_InternalArray[pos];
390 constexpr const_reference
399 return VDimension ? *(end() - 1) : *end();
402 constexpr const_reference
405 return VDimension ? *(end() - 1) : *end();
411 return &m_InternalArray[0];
417 return &m_InternalArray[0];
424 if (pos >= VDimension)
426 throw std::out_of_range(
"array::ExceptionThrowingBoundsCheck");
433 static constexpr
Self
436 return MakeFilled<Self>(value);
442 template <
unsigned int VDimension>
447 for (
unsigned int i = 0; i + 1 < VDimension; ++i)
449 os << obj[i] <<
", ";
451 if constexpr (VDimension >= 1)
453 os << obj[VDimension - 1];
461 template <
unsigned int VDimension>
468 template <
unsigned int VDimension>
472 return !(one == two);
475 template <
unsigned int VDimension>
479 return std::lexicographical_compare(one.
begin(), one.
end(), two.
begin(), two.
end());
482 template <
unsigned int VDimension>
489 template <
unsigned int VDimension>
496 template <
unsigned int VDimension>
504 template <
unsigned int VDimension>
508 std::swap(one.m_InternalArray, two.m_InternalArray);
513 template <
typename... T>
517 const auto toValueType = [](
const auto value) {
518 static_assert(std::is_integral_v<decltype(value)>,
"Each value must have an integral type!");
519 return static_cast<SizeValueType>(value);
521 return Size<
sizeof...(T)>{ { toValueType(values)... } };
constexpr const_reference front() const
constexpr SizeValueType CalculateProductOfElements() const
void SetElement(unsigned long element, SizeValueType val)
reverse_iterator rbegin()
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
ptrdiff_t difference_type
constexpr const_reference back() const
bool operator<=(const Index< VDimension > &one, const Index< VDimension > &two)
Represent a n-dimensional size (bounds) of a n-dimensional image.
void ExceptionThrowingBoundsCheck(size_type pos) const
auto MakeSize(const T... values)
itk::SizeValueType SizeValueType
std::reverse_iterator< iterator > reverse_iterator
static constexpr Self Filled(const SizeValueType value)
constexpr iterator begin()
constexpr reference front()
ITKCommon_EXPORT std::ostream & operator<<(std::ostream &out, typename AnatomicalOrientation::CoordinateEnum value)
void swap(Size &other) noexcept
const Self & operator+=(const Self &vec)
const value_type & const_reference
void Fill(SizeValueType value)
constexpr const_iterator begin() const
const_reverse_iterator rbegin() const
const_reverse_iterator rend() const
static constexpr unsigned int GetSizeDimension()
const value_type * const_iterator
constexpr reference operator[](size_type pos)
constexpr const_iterator cend() const
std::reverse_iterator< const_iterator > const_reverse_iterator
const Self & operator*=(const Self &vec)
void assign(const value_type &newValue)
const Self & operator-=(const Self &vec)
constexpr const_iterator cbegin() const
bool operator>=(const Index< VDimension > &one, const Index< VDimension > &two)
bool operator>(const Index< VDimension > &one, const Index< VDimension > &two)
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
const Self operator-(const Self &vec) const
constexpr const_reference operator[](size_type pos) const
void SetSize(const SizeValueType val[VDimension])
SizeValueType GetElement(unsigned long element) const
const Self operator*(const Self &vec) const
bool operator!=(const Index< VDimension > &one, const Index< VDimension > &two)
constexpr size_type size() const
SizeValueType m_InternalArray[VDimension]
void swap(Array< T > &a, Array< T > &b) noexcept
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
const SizeValueType * data() const
constexpr bool empty() const
const Self operator+(const Self &vec) const
itk::SizeValueType value_type
const_reference at(size_type pos) const
constexpr reference back()
constexpr const_iterator end() const
void swap(Size< VDimension > &one, Size< VDimension > &two) noexcept
constexpr unsigned int Dimension
constexpr size_type max_size() const
reference at(size_type pos)
unsigned long SizeValueType
const SizeValueType * GetSize() const