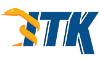 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
25 #include <type_traits>
69 template <
unsigned int VDimension = 2>
70 struct ITK_TEMPLATE_EXPORT
Index final
91 static constexpr
unsigned int Dimension = VDimension;
94 static constexpr
unsigned int
107 for (
unsigned int i = 0; i < VDimension; ++i)
109 result[i] = m_InternalArray[i] + static_cast<IndexValueType>(sz[i]);
118 for (
unsigned int i = 0; i < VDimension; ++i)
120 m_InternalArray[i] += static_cast<IndexValueType>(sz[i]);
133 for (
unsigned int i = 0; i < VDimension; ++i)
135 result[i] = m_InternalArray[i] - static_cast<IndexValueType>(sz[i]);
144 for (
unsigned int i = 0; i < VDimension; ++i)
146 m_InternalArray[i] -= static_cast<IndexValueType>(sz[i]);
158 for (
unsigned int i = 0; i < VDimension; ++i)
160 result[i] = m_InternalArray[i] + offset[i];
169 for (
unsigned int i = 0; i < VDimension; ++i)
171 m_InternalArray[i] += offset[i];
181 for (
unsigned int i = 0; i < VDimension; ++i)
183 m_InternalArray[i] -= offset[i];
195 for (
unsigned int i = 0; i < VDimension; ++i)
208 for (
unsigned int i = 0; i < VDimension; ++i)
222 for (
unsigned int i = 0; i < VDimension; ++i)
224 result[i] = m_InternalArray[i] * static_cast<IndexValueType>(vec.
m_InternalArray[i]);
234 return m_InternalArray;
244 std::copy_n(val, VDimension, m_InternalArray);
256 m_InternalArray[element] = val;
268 return m_InternalArray[element];
276 std::fill_n(begin(), size(), value);
293 static_assert(VDimension > 0,
"Error: Only positive value sized VDimension allowed");
298 template <
typename TCoordRep>
302 for (
unsigned int i = 0; i < VDimension; ++i)
304 m_InternalArray[i] = Math::Round<IndexValueType>(
point[i]);
310 template <
typename TCoordRep>
314 for (
unsigned int i = 0; i < VDimension; ++i)
316 m_InternalArray[i] = static_cast<IndexValueType>(
point[i]);
325 GetBasisIndex(
unsigned int dim);
351 std::fill_n(begin(), size(), newValue);
360 constexpr const_iterator
363 return &m_InternalArray[0];
369 return &m_InternalArray[0];
372 constexpr const_iterator
375 return &m_InternalArray[0];
378 constexpr const_iterator
381 return &m_InternalArray[VDimension];
387 return &m_InternalArray[VDimension];
390 constexpr const_iterator
393 return &m_InternalArray[VDimension];
402 const_reverse_iterator
414 const_reverse_iterator
445 ExceptionThrowingBoundsCheck(pos);
446 return m_InternalArray[pos];
452 ExceptionThrowingBoundsCheck(pos);
453 return m_InternalArray[pos];
471 return VDimension ? *(end() - 1) : *end();
477 return VDimension ? *(end() - 1) : *end();
483 return &m_InternalArray[0];
489 return &m_InternalArray[0];
495 static constexpr
Self
498 return MakeFilled<Self>(value);
506 if (pos >= VDimension)
508 throw std::out_of_range(
"array::ExceptionThrowingBoundsCheck");
514 template <
unsigned int VDimension>
524 template <
unsigned int VDimension>
529 for (
unsigned int i = 0; i + 1 < VDimension; ++i)
531 os << obj[i] <<
", ";
535 os << obj[VDimension - 1];
543 template <
unsigned int VDimension>
550 template <
unsigned int VDimension>
554 return !(one == two);
557 template <
unsigned int VDimension>
561 return std::lexicographical_compare(one.
begin(), one.
end(), two.
begin(), two.
end());
564 template <
unsigned int VDimension>
571 template <
unsigned int VDimension>
578 template <
unsigned int VDimension>
586 template <
unsigned int VDimension>
595 template <
typename... T>
599 const auto toValueType = [](
const auto value) {
600 static_assert(std::is_integral_v<decltype(value)>,
"Each value must have an integral type!");
601 return static_cast<IndexValueType>(value);
603 return Index<
sizeof...(T)>{ { toValueType(values)... } };
constexpr const_reference operator[](size_type pos) const
std::reverse_iterator< const_iterator > const_reverse_iterator
Represent a n-dimensional index in a n-dimensional image.
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
constexpr const_iterator begin() const
bool operator<=(const Index< VDimension > &one, const Index< VDimension > &two)
constexpr size_type max_size() const
Represent a n-dimensional size (bounds) of a n-dimensional image.
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
void assign(const value_type &newValue)
void CopyWithRound(const FixedArray< TCoordRep, VDimension > &point)
constexpr iterator begin()
void swap(Array< T > &a, Array< T > &b)
const_reference at(size_type pos) const
void SetIndex(const IndexValueType val[VDimension])
const Self & operator+=(const OffsetType &offset)
itk::IndexValueType IndexValueType
const Self operator*(const SizeType &vec) const
void swap(Index< VDimension > &one, Index< VDimension > &two)
const Self operator-(const OffsetType &off) const
void SetElement(unsigned long element, IndexValueType val)
const Self operator+(const SizeType &sz) const
void Fill(IndexValueType value)
const_reference front() const
itk::OffsetValueType OffsetValueType
const_reference back() const
const Self operator+(const OffsetType &offset) const
const IndexValueType * GetIndex() const
const_reverse_iterator rend() const
*par Constraints *The filter requires an image with at least two dimensions and a vector *length of at least The theory supports extension to scalar but *the implementation of the itk vector classes do not **The template parameter TRealType must be floating point(float or double) or *a user-defined "real" numerical type with arithmetic operations defined *sufficient to compute derivatives. **\par Performance *This filter will automatically multithread if run with *SetUsePrincipleComponents
reference at(size_type pos)
constexpr size_type size() const
constexpr const_iterator cend() const
const OffsetType operator-(const Self &vec) const
const value_type & const_reference
constexpr const_iterator cbegin() const
IndexValueType m_InternalArray[VDimension]
bool operator>=(const Index< VDimension > &one, const Index< VDimension > &two)
OffsetValueType m_InternalArray[VDimension]
const_reverse_iterator rbegin() const
bool operator>(const Index< VDimension > &one, const Index< VDimension > &two)
constexpr bool empty() const
ptrdiff_t difference_type
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
Simulate a standard C array with copy semantics.
void CopyWithCast(const FixedArray< TCoordRep, VDimension > &point)
static constexpr unsigned int GetIndexDimension()
static constexpr Self Filled(const IndexValueType value)
IndexValueType GetElement(unsigned long element) const
Represent a n-dimensional offset between two n-dimensional indexes of n-dimensional image.
reverse_iterator rbegin()
itk::IndexValueType value_type
bool operator!=(const Index< VDimension > &one, const Index< VDimension > &two)
auto MakeIndex(const T... values)
SizeValueType m_InternalArray[VDimension]
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
const IndexValueType * data() const
constexpr const_iterator end() const
static Self GetBasisIndex(unsigned int dim)
const Self & operator+=(const SizeType &sz)
const Self operator-(const SizeType &sz) const
const value_type * const_iterator
std::reverse_iterator< iterator > reverse_iterator
constexpr unsigned int Dimension
constexpr reference operator[](size_type pos)
void ExceptionThrowingBoundsCheck(size_type pos) const
const Self & operator-=(const SizeType &sz)
const Self & operator-=(const OffsetType &offset)