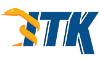 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkFixedArray_h
19 #define itkFixedArray_h
52 template <
typename TValue,
unsigned int VLength = 3>
57 static constexpr
unsigned int Length = VLength;
63 using ValueType = TValue;
74 class ConstReverseIterator;
109 return (m_Iterator - 1);
114 return *(m_Iterator - 1);
164 return (m_Iterator - 1);
169 return *(m_Iterator - 1);
224 explicit FixedArray(
const std::array<ValueType, VLength> & stdArray)
226 std::copy_n(stdArray.cbegin(), VLength, m_InternalArray);
230 template <
typename TFixedArrayValueType>
235 for (
auto & element : m_InternalArray)
237 element = static_cast<TValue>(*input++);
241 template <
typename TScalarValue>
244 std::copy_n(r, VLength, m_InternalArray);
253 template <
typename TFixedArrayValueType>
259 for (
auto & element : m_InternalArray)
261 element = static_cast<TValue>(*input++);
267 operator=(
const ValueType r[VLength]);
275 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
FixedArray);
282 ITK_GCC_SUPPRESS_Warray_bounds
286 return m_InternalArray[index];
288 constexpr const_reference
291 return m_InternalArray[index];
300 m_InternalArray[index] = value;
305 return m_InternalArray[index];
313 return m_InternalArray;
319 return m_InternalArray;
325 return m_InternalArray;
331 return m_InternalArray;
351 itkLegacyMacro(ReverseIterator rBegin();)
354 itkLegacyMacro(ConstReverseIterator rBegin()
const;)
357 itkLegacyMacro(ReverseIterator rEnd();)
360 itkLegacyMacro(ConstReverseIterator rEnd()
const;)
362 constexpr const_iterator
365 return m_InternalArray;
371 return m_InternalArray;
374 constexpr const_iterator
377 return this->cbegin();
380 constexpr const_iterator
383 return m_InternalArray + VLength;
389 return m_InternalArray + VLength;
392 constexpr const_iterator
404 const_reverse_iterator
410 const_reverse_iterator
413 return this->crbegin();
422 const_reverse_iterator
428 const_reverse_iterator
431 return this->crend();
446 Fill(
const ValueType &);
451 std::swap(m_InternalArray, other.m_InternalArray);
463 return MakeFilled<FixedArray>(value);
468 template <
typename TValue,
unsigned int VLength>
470 operator<<(std::ostream & os,
const FixedArray<TValue, VLength> & arr);
473 template <
typename TValue,
unsigned int VLength>
482 #ifndef ITK_MANUAL_INSTANTIATION
483 # include "itkFixedArray.hxx"
const_reverse_iterator rend() const
constexpr const_iterator cbegin() const noexcept
FixedArray(const TScalarValue *r)
void swap(FixedArray &other) noexcept
Represent a n-dimensional size (bounds) of a n-dimensional image.
ValueType[VLength] CArray
const ValueType * data() const
ReverseIterator operator--(int)
constexpr SizeType size() const
const ValueType & const_reference
constexpr const_iterator begin() const noexcept
A const reverse iterator through an array.
FixedArray(const FixedArray< TFixedArrayValueType, VLength > &r)
ConstReverseIterator operator++(int)
A reverse iterator through an array.
ITK_GCC_PRAGMA_POP void SetElement(unsigned int index, const_reference value)
ImageBaseType::SizeType SizeType
void swap(FixedArray< TValue, VLength > &a, FixedArray< TValue, VLength > &b) noexcept
ConstReverseIterator(const ReverseIterator &rit)
ITKCommon_EXPORT std::ostream & operator<<(std::ostream &out, typename AnatomicalOrientation::CoordinateEnum value)
const_reference GetElement(unsigned int index) const
constexpr iterator end() noexcept
FixedArray & operator=(const FixedArray< TFixedArrayValueType, VLength > &r)
constexpr const_reference operator[](unsigned int index) const
ValueType * GetDataPointer()
ReverseIterator(Iterator i)
const ValueType * ConstIterator
ConstReverseIterator operator--(int)
ConstReverseIterator(ConstIterator i)
const ValueType * GetDataPointer() const
ReverseIterator operator--()
ConstIterator operator->() const
ReverseIterator operator++(int)
const ValueType * const_pointer
constexpr const_iterator cend() const noexcept
ReverseIterator operator++()
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
ConstReverseIterator operator--()
Simulate a standard C array with copy semantics.
ValueType & operator*() const
std::reverse_iterator< iterator > reverse_iterator
constexpr const_iterator end() const noexcept
void swap(Array< T > &a, Array< T > &b) noexcept
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
const_reverse_iterator crbegin() const
ConstReverseIterator operator++()
reverse_iterator rbegin()
Iterator operator->() const
constexpr iterator begin() noexcept
ITK_GCC_PRAGMA_PUSH constexpr ITK_GCC_SUPPRESS_Warray_bounds reference operator[](unsigned int index)
const ValueType & operator*() const
static constexpr FixedArray Filled(const ValueType &value)
bool operator==(const ReverseIterator &rit) const
bool operator==(const ConstReverseIterator &rit) const
const ValueType * const_iterator
constexpr unsigned int Dimension
const_reverse_iterator crend() const
const_reverse_iterator rbegin() const
std::reverse_iterator< const_iterator > const_reverse_iterator
FixedArray(const std::array< ValueType, VLength > &stdArray)