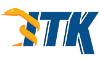 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkBinaryFunctorImageFilter_h
19 #define itkBinaryFunctorImageFilter_h
52 template <
typename TInputImage1,
typename TInputImage2,
typename TOutputImage,
typename TFunction>
91 SetInput1(
const TInputImage1 * image1);
105 GetConstant1()
const;
109 SetInput2(
const TInputImage2 * image2);
122 this->SetConstant2(ct);
124 const Input2ImagePixelType &
127 return this->GetConstant2();
133 virtual const Input2ImagePixelType &
134 GetConstant2()
const;
165 if (m_Functor != functor)
174 static constexpr
unsigned int InputImage1Dimension = TInputImage1::ImageDimension;
175 static constexpr
unsigned int InputImage2Dimension = TInputImage2::ImageDimension;
176 static constexpr
unsigned int OutputImageDimension = TOutputImage::ImageDimension;
178 #ifdef ITK_USE_CONCEPT_CHECKING
208 GenerateOutputInformation()
override;
215 #ifndef ITK_MANUAL_INSTANTIATION
216 # include "itkBinaryFunctorImageFilter.hxx"
TInputImage2 Input2ImageType
SmartPointer< Self > Pointer
Decorates any "simple" data type (data types without smart pointers) with a DataObject API.
typename Input2ImageType::RegionType Input2ImageRegionType
SmartPointer< const Self > ConstPointer
void SetFunctor(const FunctorType &functor)
typename Input1ImageType::PixelType Input1ImagePixelType
typename OutputImageType::Pointer OutputImagePointer
Base class for filters that take an image as input and overwrite that image as the output.
typename Input2ImageType::ConstPointer Input2ImagePointer
Implements pixel-wise generic operation of two images, or of an image and a constant.
TInputImage1 Input1ImageType
Base class for all process objects that output image data.
FunctorType & GetFunctor()
ImageBaseType::RegionType RegionType
Functor::Add2< typename TInputImage1::PixelType, typename TInputImage2::PixelType, typename TOutputImage::PixelType > FunctorType
typename Input1ImageType::RegionType Input1ImageRegionType
const FunctorType & GetFunctor() const
typename Input2ImageType::PixelType Input2ImagePixelType
void SetConstant(Input2ImagePixelType ct)
typename OutputImageType::RegionType OutputImageRegionType
#define itkConceptMacro(name, concept)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
typename OutputImageType::PixelType OutputImagePixelType
const Input2ImagePixelType & GetConstant() const
typename Input1ImageType::ConstPointer Input1ImagePointer
TOutputImage OutputImageType