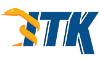 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkBinaryNotImageFilter_h
19 #define itkBinaryNotImageFilter_h
57 template <
typename TPixel>
85 template <
typename TImage>
109 itkGetConstMacro(ForegroundValue,
PixelType);
115 itkGetConstMacro(BackgroundValue,
PixelType);
133 os << indent <<
"ForegroundValue: " << static_cast<PixelPrintType>(
m_ForegroundValue) << std::endl;
135 os << indent <<
"BackgroundValue: " << static_cast<PixelPrintType>(
m_BackgroundValue) << std::endl;
Implements the BinaryNot logical operator pixel-wise between two images.
Implements pixel-wise generic operation on one image.
typename TImage::PixelType PixelType
ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(BinaryNot)
static constexpr T NonpositiveMin()
~BinaryNotImageFilter() override=default
Control indentation during Print() invocation.
Light weight base class for most itk classes.
void GenerateData() override
void PrintSelf(std::ostream &os, Indent indent) const override
void GenerateData() override
TPixel operator()(const TPixel &A) const
Define additional traits for native types such as int or float.
static constexpr T max(const T &)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
PixelType m_ForegroundValue
void PrintSelf(std::ostream &os, Indent indent) const override
FunctorType & GetFunctor()
bool operator==(const BinaryNot &) const
PixelType m_BackgroundValue