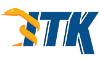 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkBinaryThresholdProjectionImageFilter_h
19 #define itkBinaryThresholdProjectionImageFilter_h
52 template <
typename TInputPixel,
typename TOutputPixel>
95 template <
typename TInputImage,
typename TOutputImage>
100 Function::BinaryThresholdAccumulator<typename TInputImage::PixelType, typename TOutputImage::PixelType>>
148 #ifdef ITK_USE_CONCEPT_CHECKING
172 os << indent <<
"ForegroundValue: " << static_cast<InputPixelPrintType>(
m_ForegroundValue) << std::endl;
176 os << indent <<
"BackgroundValue: " << static_cast<OutputPixelPrintType>(
m_BackgroundValue) << std::endl;
178 os << indent <<
"ThresholdValue: " << static_cast<InputPixelPrintType>(
m_ThresholdValue) << std::endl;
TAccumulator AccumulatorType
void PrintSelf(std::ostream &os, Indent indent) const override
static constexpr T NonpositiveMin()
TInputPixel m_ThresholdValue
Control indentation during Print() invocation.
OutputPixelType m_ForegroundValue
Implements an accumulation of an image along a selected direction.
Base class for all process objects that output image data.
void operator()(const TInputPixel &input)
typename OutputImageType::PixelType OutputPixelType
BinaryThresholdProjectionImageFilter()
BinaryThresholdAccumulator(SizeValueType)
InputPixelType m_ThresholdValue
TInputImage InputImageType
void PrintSelf(std::ostream &os, Indent indent) const override
AccumulatorType NewAccumulator(SizeValueType size) const override
Define additional traits for native types such as int or float.
static constexpr T max(const T &)
~BinaryThresholdAccumulator()=default
#define itkConceptMacro(name, concept)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
TOutputPixel m_BackgroundValue
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
BinaryThreshold projection.
~BinaryThresholdProjectionImageFilter() override=default
OutputPixelType m_BackgroundValue
typename InputImageType::PixelType InputPixelType
TOutputPixel m_ForegroundValue
unsigned long SizeValueType
TOutputImage OutputImageType