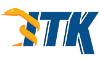 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkConformalFlatteningMeshFilter_h
19 #define itkConformalFlatteningMeshFilter_h
26 #include "vnl/vnl_sparse_matrix.h"
46 template <
typename TInputMesh,
typename TOutputMesh>
79 static constexpr
unsigned int InputPointDimension = TInputMesh::PointDimension;
80 static constexpr
unsigned int OutputPointDimension = TOutputMesh::PointDimension;
88 using CellType =
typename InputMeshType::CellType;
118 PrintSelf(std::ostream & os,
Indent indent)
const override;
122 GenerateData()
override;
130 unsigned int m_PolarCellIdentifier{};
133 bool m_MapToSphere{};
141 #ifndef ITK_MANUAL_INSTANTIATION
142 # include "itkConformalFlatteningMeshFilter.hxx"
SmartPointer< Self > Pointer
SmartPointer< const Self > ConstPointer
typename OutputMeshType::Pointer OutputMeshPointer
ImageBaseType::PointType PointType
Control indentation during Print() invocation.
Light weight base class for most itk classes.
MeshToMeshFilter is the base class for all process objects that output mesh data, and require mesh da...
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
TOutputMesh OutputMeshType