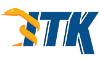 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkFixedCenterOfRotationAffineTransform_h
19 #define itkFixedCenterOfRotationAffineTransform_h
33 template <
typename TParametersValueType =
double,
unsigned int VDimension = 3>
54 static constexpr
unsigned int InputSpaceDimension = VDimension;
55 static constexpr
unsigned int OutputSpaceDimension = VDimension;
56 static constexpr
unsigned int SpaceDimension = VDimension;
57 static constexpr
unsigned int ParametersDimension = VDimension * (VDimension + 2);
60 using typename Superclass::ParametersType;
61 using typename Superclass::FixedParametersType;
89 return this->GetCenter();
98 this->SetMatrix(matrix);
105 return this->GetMatrix();
112 this->SetTranslation(offset);
119 return this->GetTranslation();
124 #if !defined(ITK_LEGACY_REMOVE)
126 const OutputVectorType & offset);
137 #ifndef ITK_MANUAL_INSTANTIATION
138 # include "itkFixedCenterOfRotationAffineTransform.hxx"
A templated class holding a n-Dimensional covariant vector.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Array2D class representing a 2D array.