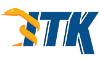 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkCovariantVector_h
19 #define itkCovariantVector_h
23 #include "vnl/vnl_vector_ref.h"
69 template <
typename T,
unsigned int VVectorDimension = 3>
86 static constexpr
unsigned int Dimension = VVectorDimension;
98 return VVectorDimension;
103 SetVnlVector(
const vnl_vector<T> &);
111 GetVnlVector()
const;
124 template <
typename TVectorValueType>
134 template <
typename TCovariantVectorValueType>
138 BaseArray::operator=(r);
145 operator=(
const ValueType r[VVectorDimension]);
148 template <
typename Tt>
152 for (
unsigned int i = 0; i < VVectorDimension; ++i)
154 (*this)[i] = static_cast<ValueType>((*
this)[i] * value);
161 template <
typename Tt>
165 for (
unsigned int i = 0; i < VVectorDimension; ++i)
167 (*this)[i] = static_cast<ValueType>((*
this)[i] / value);
175 operator+=(
const Self & vec);
179 operator-=(
const Self & vec);
210 for (
unsigned int i = 0; i < VVectorDimension; ++i)
212 result[i] = static_cast<ValueType>((*
this)[i] * val);
219 template <
typename Tt>
225 for (
unsigned int i = 0; i < VVectorDimension; ++i)
227 result[i] = static_cast<ValueType>((*
this)[i] / val);
240 return VVectorDimension;
249 GetSquaredNorm()
const;
253 template <
typename TCoordRepB>
257 for (
unsigned int i = 0; i < VVectorDimension; ++i)
259 (*this)[i] = static_cast<T>(pa[i]);
267 template <
typename T,
unsigned int VVectorDimension>
270 return v.operator*(scalar);
275 template <
typename T,
unsigned int VVectorDimension>
279 return covariant.operator*(contravariant);
282 ITKCommon_EXPORT
void
283 CrossProduct(CovariantVector<double, 3> &,
const Vector<double, 3> &,
const Vector<double, 3> &);
285 ITKCommon_EXPORT
void
286 CrossProduct(CovariantVector<float, 3> &,
const Vector<float, 3> &,
const Vector<float, 3> &);
288 ITKCommon_EXPORT
void
289 CrossProduct(CovariantVector<int, 3>,
const Vector<int, 3> &,
const Vector<int, 3> &);
292 template <
typename T,
unsigned int VVectorDimension>
311 #ifndef ITK_MANUAL_INSTANTIATION
312 # include "itkCovariantVector.hxx"
void CastFrom(const CovariantVector< TCoordRepB, VVectorDimension > &pa)
CovariantVector(const ValueType r[Dimension])
const Self & operator/=(const Tt &value)
CovariantVector(const CovariantVector< TVectorValueType, VVectorDimension > &r)
Self operator/(const Tt &val) const
CovariantVector< T, VVectorDimension > operator*(const T &scalar, const CovariantVector< T, VVectorDimension > &v)
Self operator*(const ValueType &val) const
A templated class holding a n-Dimensional vector.
ConstNeighborhoodIterator< TImage > operator-(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
static unsigned int GetNumberOfComponents()
typename NumericTraits< ValueType >::RealType RealValueType
static unsigned int GetCovariantVectorDimension()
ITKCommon_EXPORT void CrossProduct(CovariantVector< double, 3 > &, const Vector< double, 3 > &, const Vector< double, 3 > &)
const Self & operator*=(const Tt &value)
Self & operator=(const CovariantVector< TCovariantVectorValueType, VVectorDimension > &r)
Simulate a standard C array with copy semantics.
A templated class holding a n-Dimensional covariant vector.
void swap(Array< T > &a, Array< T > &b) noexcept
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
void swap(FixedArray &other)
ConstNeighborhoodIterator< TImage > operator+(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
constexpr unsigned int Dimension