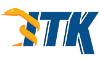 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
23 #include "vnl/vnl_vector_ref.h"
61 template <
typename T,
unsigned int VVectorDimension = 3>
75 static constexpr
unsigned int Dimension = VVectorDimension;
90 return VVectorDimension;
96 SetVnlVector(
const vnl_vector<T> &);
104 GetVnlVector()
const;
110 #if !defined(ITK_LEGACY_REMOVE)
115 Vector(
const ValueType & r);
120 explicit Vector(
const ValueType & r);
123 Vector(std::nullptr_t) =
delete;
127 template <
typename TVectorValueType>
134 template <
typename TVectorValueType>
141 explicit Vector(
const std::array<ValueType, VVectorDimension> & stdArray)
146 template <
typename TVectorValueType>
150 BaseArray::operator=(r);
156 operator=(
const ValueType r[VVectorDimension]);
159 template <
typename Tt>
163 for (
unsigned int i = 0; i < VVectorDimension; ++i)
165 (*this)[i] = static_cast<ValueType>((*
this)[i] * value);
172 template <
typename Tt>
176 for (
unsigned int i = 0; i < VVectorDimension; ++i)
178 (*this)[i] = static_cast<ValueType>((*
this)[i] / value);
186 operator+=(
const Self & vec);
190 operator-=(
const Self & vec);
217 for (
unsigned int i = 0; i < VVectorDimension; ++i)
219 result[i] = static_cast<ValueType>((*
this)[i] * value);
226 template <
typename Tt>
232 for (
unsigned int i = 0; i < VVectorDimension; ++i)
234 result[i] = static_cast<ValueType>((*
this)[i] / value);
249 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
257 GetSquaredNorm()
const;
263 return VVectorDimension;
274 this->operator[](c) = v;
279 template <
typename TCoordinateB>
283 for (
unsigned int i = 0; i < VVectorDimension; ++i)
285 (*this)[i] = static_cast<T>(pa[i]);
290 template <
typename TCoordinateB>
294 for (
unsigned int i = 0; i < VVectorDimension; ++i)
296 r[i] = static_cast<TCoordinateB>((*
this)[i]);
304 template <
typename T,
unsigned int VVectorDimension>
305 inline Vector<T, VVectorDimension>
308 return v.operator*(scalar);
312 template <
typename T,
unsigned int VVectorDimension>
314 operator<<(std::ostream & os,
const Vector<T, VVectorDimension> & vct);
317 template <
typename T,
unsigned int VVectorDimension>
319 operator>>(std::istream & is, Vector<T, VVectorDimension> & vct);
321 ITKCommon_EXPORT Vector<double, 3>
322 CrossProduct(
const Vector<double, 3> &,
const Vector<double, 3> &);
324 ITKCommon_EXPORT Vector<float, 3>
325 CrossProduct(
const Vector<float, 3> &,
const Vector<float, 3> &);
327 ITKCommon_EXPORT Vector<int, 3>
328 CrossProduct(
const Vector<int, 3> &,
const Vector<int, 3> &);
331 template <
typename T,
unsigned int VVectorDimension>
340 template <
typename TValue,
typename... TVariadic>
342 MakeVector(
const TValue firstValue,
const TVariadic... otherValues)
344 static_assert(std::conjunction_v<std::is_same<TVariadic, TValue>...>,
345 "The other values should have the same type as the first value.");
347 constexpr
unsigned int dimension{ 1 +
sizeof...(TVariadic) };
348 const std::array<TValue, dimension> stdArray{ { firstValue, otherValues... } };
354 #ifndef ITK_MANUAL_INSTANTIATION
355 # include "itkVector.hxx"
const Self & operator/=(const Tt &value)
const Self & operator*=(const Tt &value)
CovariantVector< T, VVectorDimension > operator*(const T &scalar, const CovariantVector< T, VVectorDimension > &v)
auto MakeVector(const TValue firstValue, const TVariadic... otherValues)
Self operator*(const ValueType &value) const
A templated class holding a n-Dimensional vector.
ConstNeighborhoodIterator< TImage > operator-(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
Vector(const std::array< ValueType, VVectorDimension > &stdArray)
Vector(const ValueType r[Dimension])
ITKCommon_EXPORT std::ostream & operator<<(std::ostream &out, typename AnatomicalOrientation::CoordinateEnum value)
Self operator/(const Tt &value) const
typename NumericTraits< ValueType >::RealType RealValueType
static unsigned int GetNumberOfComponents()
void SetNthComponent(int c, const ComponentType &v)
ITKCommon_EXPORT void CrossProduct(CovariantVector< double, 3 > &, const Vector< double, 3 > &, const Vector< double, 3 > &)
void CastFrom(const Vector< TCoordinateB, VVectorDimension > &pa)
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
Simulate a standard C array with copy semantics.
static unsigned int GetVectorDimension()
void swap(Array< T > &a, Array< T > &b) noexcept
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Vector & operator=(const Vector< TVectorValueType, VVectorDimension > &r)
ConstNeighborhoodIterator< TImage > operator+(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
Vector(const Vector< TVectorValueType, VVectorDimension > &r)
bool operator==(const Self &v) const
Vector(const TVectorValueType r[Dimension])
constexpr unsigned int Dimension
std::istream & operator>>(std::istream &is, Point< T, VPointDimension > &vct)