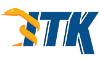 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkHDF5TransformIO_h
19 #define itkHDF5TransformIO_h
21 #include "ITKIOTransformHDF5Export.h"
85 template <
typename TParametersValueType>
94 using typename Superclass::TransformType;
95 using typename Superclass::TransformPointer;
96 using typename Superclass::TransformListType;
112 CanReadFile(
const char *)
override;
117 CanWriteFile(
const char *)
override;
136 ReadParameters(
const std::string & DataSetName)
const;
138 ReadFixedParameters(
const std::string & DataSetName)
const;
143 WriteParameters(
const std::string & name,
const ParametersType & parameters);
145 WriteFixedParameters(
const std::string & name,
const FixedParametersType & fixedParameters);
150 WriteString(
const std::string & path,
const std::string & value);
152 WriteString(
const std::string & path,
const char * s);
154 WriteOneTransform(
const int transformIndex,
const TransformType * curTransform);
164 GetH5TypeFromString()
const;
167 std::string ITKIOTransformHDF5_EXPORT
177 #endif // itkHDF5TransformIO_h
180 #ifndef ITK_TEMPLATE_EXPLICIT_HDF5TransformIO
189 #if defined(ITKIOTransformHDF5_EXPORTS)
191 # define ITKIOTransformHDF5_EXPORT_EXPLICIT ITK_FORWARD_EXPORT
194 # define ITKIOTransformHDF5_EXPORT_EXPLICIT ITKIOTransformHDF5_EXPORT
198 ITK_GCC_PRAGMA_DIAG_PUSH()
199 ITK_GCC_PRAGMA_DIAG(ignored
"-Wattributes")
204 ITK_GCC_PRAGMA_DIAG_POP()
207 #undef ITKIOTransformHDF5_EXPORT_EXPLICIT
static const std::string transformTypeName
static const std::string transformParamsNameCorrected
static const std::string transformFixedName
std::string ITKIOTransformHDF5_EXPORT GetTransformName(int)
static const std::string transformParamsName
Light weight base class for most itk classes.
static const std::string transformParamsNameMisspelled
static const std::string OSName
static const std::string transformFixedNameMisspelled
static const std::string transformGroupName
Secondary bass class of HDF5CommonPathNames common between templates.
static const std::string OSVersion
static const std::string transformFixedNameCorrected
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
static const std::string ItkVersion
Base class for most ITK classes.
template class ITKIOTransformHDF5_EXPORT_EXPLICIT HDF5TransformIOTemplate< float >
template class ITKIOTransformHDF5_EXPORT_EXPLICIT HDF5TransformIOTemplate< double >
static const std::string HDFVersion