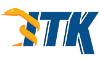 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkHessianRecursiveGaussianImageFilter_h
19 #define itkHessianRecursiveGaussianImageFilter_h
42 template <
typename TInputImage,
43 typename TOutputImage =
44 Image<SymmetricSecondRankTensor<typename NumericTraits<typename TInputImage::PixelType>::RealType,
45 TInputImage::ImageDimension>,
46 TInputImage::ImageDimension>>
64 static constexpr
unsigned int ImageDimension = TInputImage::ImageDimension;
67 static constexpr
unsigned int NumberOfSmoothingFilters =
68 (TInputImage::ImageDimension > 2) ? (TInputImage::ImageDimension - 2) : (0);
126 SetNormalizeAcrossScale(
bool normalize);
127 itkGetConstMacro(NormalizeAcrossScale,
bool);
128 itkBooleanMacro(NormalizeAcrossScale);
137 GenerateInputRequestedRegion()
override;
139 #ifdef ITK_USE_CONCEPT_CHECKING
150 PrintSelf(std::ostream & os,
Indent indent)
const override;
154 GenerateData()
override;
158 EnlargeOutputRequestedRegion(
DataObject * output)
override;
167 bool m_NormalizeAcrossScale{};
171 #ifndef ITK_MANUAL_INSTANTIATION
172 # include "itkHessianRecursiveGaussianImageFilter.hxx"
typename DerivativeFilterBType::Pointer DerivativeFilterBPointer
SmartPointer< Self > Pointer
Presents an image as being composed of the N-th element of its pixels.
typename OutputImageType::Pointer OutputImagePointer
Control indentation during Print() invocation.
typename OutputImageType::PixelType OutputPixelType
typename PixelTraits< OutputPixelType >::ValueType OutputComponentType
typename TInputImage::PixelType PixelType
Base class for filters that take an image as input and produce an image as output.
Base class for all process objects that output image data.
typename DerivativeFilterAType::Pointer DerivativeFilterAPointer
typename OutputImageAdaptorType::Pointer OutputImageAdaptorPointer
TInputImage InputImageType
Computes the Hessian matrix of an image by convolution with the Second and Cross derivatives of a Gau...
Define additional traits for native types such as int or float.
typename TPixelType::ValueType ValueType
#define itkConceptMacro(name, concept)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
typename NumericTraits< PixelType >::RealType RealType
std::vector< GaussianFilterPointer > GaussianFiltersArray
Templated n-dimensional image class.
Base class for computing IIR convolution with an approximation of a Gaussian kernel.
typename GaussianFilterType::Pointer GaussianFilterPointer
TOutputImage OutputImageType
Base class for all data objects in ITK.