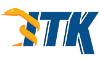 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkImageToNeighborhoodSampleAdaptor_h
19 #define itkImageToNeighborhoodSampleAdaptor_h
54 template <
typename TImage,
typename TBoundaryCondition>
56 :
public ListSample<std::vector<ConstNeighborhoodIterator<TImage, TBoundaryCondition>>>
100 using ValueType =
typename MeasurementVectorType::value_type;
103 using typename Superclass::AbsoluteFrequencyType;
104 using typename Superclass::TotalAbsoluteFrequencyType;
105 using typename Superclass::MeasurementVectorSizeType;
106 using typename Superclass::InstanceIdentifier;
110 SetImage(
const TImage * image);
133 SetUseImageRegion(
const bool flag);
136 itkGetConstMacro(UseImageRegion,
bool);
139 itkBooleanMacro(UseImageRegion);
144 Size()
const override;
156 GetTotalFrequency()
const override;
195 return this->m_MeasurementVectorCache;
201 return m_InstanceIdentifier;
207 ++(m_MeasurementVectorCache[0]);
208 ++m_InstanceIdentifier;
224 this->m_MeasurementVectorCache.clear();
225 this->m_MeasurementVectorCache.push_back(iter);
226 m_InstanceIdentifier = iid;
262 this->ConstIterator::operator=(iter);
290 Iterator iter(nIterator, m_Region.GetNumberOfPixels());
322 PrintSelf(std::ostream & os,
Indent indent)
const override;
331 bool m_UseImageRegion{
true };
338 template <
typename TImage,
typename TBoundaryCondition>
344 #ifndef ITK_MANUAL_INSTANTIATION
345 # include "itkImageToNeighborhoodSampleAdaptor.hxx"
SmartPointer< Self > Pointer
typename ImageType::OffsetType OffsetType
ConstIterator & operator=(const ConstIterator &iter)
SmartPointer< const Self > ConstPointer
Iterator & operator=(const Iterator &iter)
typename ImageType::PixelType PixelType
Represent a n-dimensional size (bounds) of a n-dimensional image.
ConstIterator End() const
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
bool operator==(const ConstIterator &it) const
ConstIterator(const ConstIterator &iter)
typename MeasurementVectorType::value_type ValueType
Iterator(const Iterator &iter)
typename NeighborhoodIteratorType::NeighborhoodType NeighborhoodType
typename ImageType::SizeType SizeType
typename ImageType::PixelContainerConstPointer PixelContainerConstPointer
NumericTraits< AbsoluteFrequencyType >::AccumulateType TotalAbsoluteFrequencyType
A light-weight container object for storing an N-dimensional neighborhood of values.
ImageBaseType::SizeType SizeType
typename ImageType::Pointer ImagePointer
This class is the native implementation of the a Sample with an STL container.
AbsoluteFrequencyType GetFrequency() const
typename NeighborhoodIteratorType::RadiusType NeighborhoodRadiusType
Control indentation during Print() invocation.
typename MeasurementVectorTraits::InstanceIdentifier InstanceIdentifier
typename NeighborhoodIteratorType::IndexType NeighborhoodIndexType
ImageBaseType::IndexType IndexType
This class provides ListSample interface to ITK Image.
typename RegionType::OffsetTableType OffsetTableType
ConstIterator(const ImageToNeighborhoodSampleAdaptor *adaptor)
ValueType MeasurementType
typename std::vector< ConstNeighborhoodIterator< TImage, TBoundaryCondition > > MeasurementVectorType
MeasurementVectorTraits::AbsoluteFrequencyType AbsoluteFrequencyType
ImageBaseType::RegionType RegionType
InstanceIdentifier m_InstanceIdentifier
InstanceIdentifier GetInstanceIdentifier() const
A multi-dimensional iterator templated over image type that walks pixels within a region and is speci...
MeasurementVectorType m_MeasurementVectorCache
ConstIterator & operator++()
typename ImageType::IndexType IndexType
const MeasurementVectorType & GetMeasurementVector() const
Defines iteration of a local N-dimensional neighborhood of pixels across an itk::Image.
typename NeighborhoodIteratorType::SizeType NeighborhoodSizeType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Const version of NeighborhoodIterator, defining iteration of a local N-dimensional neighborhood of pi...
typename ImageType::OffsetValueType OffsetValueType
Base class for most ITK classes.
typename ImageType::RegionType RegionType
ConstIterator(NeighborhoodIteratorType iter, InstanceIdentifier iid)
ITK_ITERATOR_VIRTUAL void GoToEnd() ITK_ITERATOR_FINAL
typename ImageType::ConstPointer ImageConstPointer
ConstIterator Begin() const
Base class for all data objects in ITK.
ITK_ITERATOR_VIRTUAL void GoToBegin() ITK_ITERATOR_FINAL
Iterator(NeighborhoodIteratorType iter, InstanceIdentifier iid)