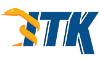 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkLabelOverlayFunctor_h
19 #define itkLabelOverlayFunctor_h
47 template <
typename TInputPixel,
typename TLabel,
typename TRGBPixel>
62 operator()(
const TInputPixel & p1,
const TLabel & p2)
const
71 auto p = static_cast<typename TRGBPixel::ValueType>(p1);
83 const double p1_blend = p1 * (1.0 -
m_Opacity);
84 rgbPixel[0] = static_cast<typename TRGBPixel::ValueType>(opaque[0] *
m_Opacity + p1_blend);
85 rgbPixel[1] = static_cast<typename TRGBPixel::ValueType>(opaque[1] *
m_Opacity + p1_blend);
86 rgbPixel[2] = static_cast<typename TRGBPixel::ValueType>(opaque[2] *
m_Opacity + p1_blend);
131 AddColor(
unsigned char r,
unsigned char g,
unsigned char b)
typename OutputImagePixelType ::ComponentType ComponentType
TRGBPixel operator()(const TInputPixel &p1, const TLabel &p2) const
void SetOpacity(double opacity)
Functor for applying a colormap to a label image and combine it with a grayscale image.
Functor::LabelToRGBFunctor< TLabel, TRGBPixel > m_RGBFunctor
Functor for converting labels into RGB triplets.
bool ExactlyEquals(const TInput1 &x1, const TInput2 &x2)
Return the result of an exact comparison between two scalar values of potentially different types.
static void SetLength(T &m, const unsigned int s)
ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(LabelOverlayFunctor)
~LabelOverlayFunctor()=default
bool operator==(const LabelOverlayFunctor &other) const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
void SetBackgroundValue(TLabel v)
void AddColor(unsigned char r, unsigned char g, unsigned char b)
unsigned int GetNumberOfColors() const