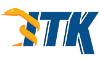 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkLevelSetNode_h
19 #define itkLevelSetNode_h
44 template <
typename TPixel,
unsigned int VSetDimension = 2>
bool operator<=(const Self &node) const
LevelSetNode(const Self &node)
bool operator>=(const Self &node) const
void SetValue(const PixelType &input)
bool operator<(const Self &node) const
Represent a node in a level set.
void Fill(IndexValueType value)
Index< VSetDimension > IndexType
static constexpr unsigned int SetDimension
Self & operator=(const Self &rhs)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
const IndexType & GetIndex() const
void SetIndex(const IndexType &input)
bool operator>(const Self &node) const
const PixelType & GetValue() const