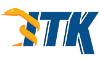 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkLogicOpsFunctors_h
19 #define itkLogicOpsFunctors_h
58 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
66 m_BackgroundValue = TOutput{};
78 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
83 m_ForegroundValue = FG;
88 m_BackgroundValue = BG;
94 return (m_ForegroundValue);
99 return (m_BackgroundValue);
117 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
129 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
136 return this->m_ForegroundValue;
138 return this->m_BackgroundValue;
151 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
163 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
170 return this->m_ForegroundValue;
172 return this->m_BackgroundValue;
186 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
198 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
205 return this->m_ForegroundValue;
207 return this->m_BackgroundValue;
221 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
233 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
240 return this->m_ForegroundValue;
242 return this->m_BackgroundValue;
256 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
268 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
275 return this->m_ForegroundValue;
277 return this->m_BackgroundValue;
291 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
303 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
310 return this->m_ForegroundValue;
312 return this->m_BackgroundValue;
322 template <
typename TInput,
typename TOutput = TInput>
332 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
NOT);
339 return this->m_ForegroundValue;
341 return this->m_BackgroundValue;
350 template <
typename TInput1,
typename TInput2,
typename TInput3,
typename TOutput>
363 operator()(
const TInput1 & A,
const TInput2 & B,
const TInput3 & C)
const
367 return static_cast<TOutput>(B);
370 return static_cast<TOutput>(C);
Functor for > operation on images and constants.
TOutput operator()(const TInput1 &A, const TInput2 &B) const
Functor for >= operation on images and constants.
bool operator==(const Self &) const
TOutput operator()(const TInput1 &A, const TInput2 &B, const TInput3 &C) const
TOutput operator()(const TInput &A) const
TOutput GetForegroundValue() const
TOutput operator()(const TInput1 &A, const TInput2 &B) const
Base class for some logic functors. Provides the Foreground and background setting methods.
Unary logical NOT functor.
bool ExactlyEquals(const TInput1 &x1, const TInput2 &x2)
Return the result of an exact comparison between two scalar values of potentially different types.
bool NotExactlyEquals(const TInput1 &x1, const TInput2 &x2)
bool operator==(const Self &) const
bool operator==(const Self &) const
bool operator==(const Self &) const
void SetForegroundValue(const TOutput &FG)
TOutput m_ForegroundValue
bool operator==(const Self &) const
bool operator==(const NOT &) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const
TOutput m_BackgroundValue
Functor for <= operation on images and constants.
Functor for == operation on images and constants.
bool operator==(const Self &) const
Return argument 2 if argument 1 is false, and argument 3 otherwise.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
TOutput operator()(const TInput1 &A, const TInput2 &B) const
Functor for != operation on images and constants.
TOutput operator()(const TInput1 &A, const TInput2 &B) const
TOutput GetBackgroundValue() const
bool operator==(const Self &) const
Functor for < operation on images and constants.
void SetBackgroundValue(const TOutput &BG)
bool operator==(const TernaryOperator &) const