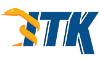 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkMovingHistogramImageFilterBase_h
19 #define itkMovingHistogramImageFilterBase_h
90 template <
typename TInputImage,
typename TOutputImage,
typename TKernel>
116 using typename Superclass::OutputImageRegionType;
120 static constexpr
unsigned int ImageDimension = TInputImage::ImageDimension;
133 using OffsetMapType =
typename std::map<OffsetType, OffsetListType, Functor::LexicographicCompare>;
137 SetKernel(
const KernelType & kernel)
override;
146 PrintSelf(std::ostream & os,
Indent indent)
const override;
149 GetDirAndOffset(
const IndexType LineStart,
153 int & LineDirection);
172 m_Dimension = dimension;
204 #ifndef ITK_MANUAL_INSTANTIATION
205 # include "itkMovingHistogramImageFilterBase.hxx"
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
typename TOutputImage::PixelType OutputPixelType
typename KernelType::ConstIterator KernelIteratorType
DirectionCost(int dimension, int count)
ImageBaseType::SizeType SizeType
Control indentation during Print() invocation.
typename TInputImage::SizeType RadiusType
typename TInputImage::OffsetType OffsetType
ImageBaseType::IndexType IndexType
Base class for all process objects that output image data.
typename TInputImage::IndexType IndexType
typename TImageType ::PixelType PixelType
Implements a generic moving histogram algorithm.
ImageBaseType::RegionType RegionType
TInputImage InputImageType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
typename std::map< OffsetType, OffsetListType, Functor::LexicographicCompare > OffsetMapType
typename TInputImage::SizeType SizeType
A base class for all the filters working on an arbitrary shaped neighborhood.
typename std::list< OffsetType > OffsetListType
unsigned long SizeValueType
TOutputImage OutputImageType
typename TInputImage::RegionType RegionType