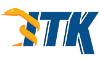 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkNeighborhoodAllocator_h
19 #define itkNeighborhoodAllocator_h
43 template <
typename TPixel>
67 m_Data = make_unique_for_overwrite<TPixel[]>(n);
93 ,
m_Data{ std::move(other.m_Data) }
95 other.m_ElementCount = 0;
121 m_Data = std::move(other.m_Data);
122 other.m_ElementCount = 0;
169 m_Data = make_unique_for_overwrite<TPixel[]>(n);
192 template <
typename TPixel>
193 inline std::ostream &
196 o <<
"NeighborhoodAllocator { this = " << &a <<
", begin = " << static_cast<const void *>(a.
begin())
197 <<
", size=" << a.
size() <<
" }";
203 template <
typename TPixel>
207 const unsigned int size = lhs.
size();
208 return (size == rhs.
size()) && ((size == 0) || std::equal(lhs.
begin(), lhs.
end(), rhs.
begin()));
212 template <
typename TPixel>
216 return !(lhs == rhs);
TPixel & operator[](unsigned int i)
void Allocate(unsigned int n)
unsigned int m_ElementCount
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
NeighborhoodAllocator(Self &&other) noexcept
void set_size(unsigned int n)
const TPixel * data() const noexcept
auto make_unique_for_overwrite(const vcl_size_t numberOfElements)
std::unique_ptr< TPixel[]> m_Data
const_iterator begin() const
unsigned int size() const
Self & operator=(const Self &other)
Self & operator=(Self &&other) noexcept
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
const ImageType ::InternalPixelType * * const_iterator
bool operator!=(const Index< VDimension > &one, const Index< VDimension > &two)
NeighborhoodAllocator()=default
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
const TPixel & operator[](unsigned int i) const
const_iterator end() const
NeighborhoodAllocator(const Self &other)
~NeighborhoodAllocator()=default
ImageType ::InternalPixelType * * iterator
A memory allocator for use as the default allocator type in Neighborhood.