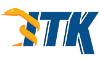 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkNumericTraitsVectorPixel_h
19 #define itkNumericTraitsVectorPixel_h
30 template <
typename T,
unsigned int D>
136 for (
unsigned int i = 0; i <
GetLength(a); ++i)
150 for (
unsigned int i = 0; i <
GetLength(a); ++i)
164 for (
unsigned int i = 0; i <
GetLength(a); ++i)
178 for (
unsigned int i = 0; i <
GetLength(a); ++i)
188 static constexpr
bool IsSigned = std::is_signed_v<ValueType>;
189 static constexpr
bool IsInteger = std::is_integral_v<ValueType>;
200 itkGenericExceptionMacro(
"Cannot set the size of a Vector of length " << D <<
" to " << s);
226 template <
typename TArray>
230 for (
unsigned int i = 0; i < D; ++i)
244 #endif // itkNumericTraitsVectorPixel_h
typename NumericTraits< T >::PrintType ElementPrintType
static unsigned int GetLength(const Vector< T, D > &)
static bool IsNonpositive(const Self &a)
static bool IsPositive(const Self &a)
typename NumericTraits< T >::AbsType ElementAbsType
static void AssignToArray(const Self &v, MeasurementVectorType &mv)
static constexpr T NonpositiveMin()
A templated class holding a n-Dimensional vector.
static const Self OneValue()
typename NumericTraits< T >::FloatType ElementFloatType
static const Self min(const Self &)
static const Self OneValue(const Self &)
static const Self ZeroValue(const Self &)
static constexpr bool IsSigned
static const Self max(const Self &)
static const Self ITKCommon_EXPORT Zero
static constexpr bool IsInteger
static const Self NonpositiveMin()
static const Self ZeroValue()
static unsigned int GetLength()
Define additional traits for native types such as int or float.
static const Self NonpositiveMin(const Self &)
static bool IsNegative(const Self &a)
typename NumericTraits< T >::AccumulateType ElementAccumulateType
static const Self ITKCommon_EXPORT One
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
static unsigned int GetLength()
typename NumericTraits< T >::RealType ElementRealType
static constexpr bool IsComplex
ElementRealType ScalarRealType
static void AssignToArray(const Self &v, TArray &mv)
void Fill(const ValueType &)
static void SetLength(Vector< T, D > &m, const unsigned int s)
static bool IsNonnegative(const Self &a)