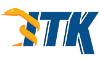 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkPathIterator_h
19 #define itkPathIterator_h
67 template <
typename TImage,
typename TPath>
79 static constexpr
unsigned int ImageIteratorDimension = TImage::ImageDimension;
109 const_cast<ImageType *>(this->m_Image.GetPointer())->SetPixel(this->m_CurrentImageIndex, value);
119 return this->GetImage()->GetPixel(this->m_ImageIndex);
125 operator=(
const Self & it);
128 PathIterator(ImageType * imagePtr,
const PathType * pathPtr);
135 #ifndef ITK_MANUAL_INSTANTIATION
136 # include "itkPathIterator.hxx"
PathIterator iterates (traces) over a path through an image.
void Set(const PixelType &value)
typename TImage::PixelContainer PixelContainer
ImageBaseType::SizeType SizeType
typename PathType::InputType PathInputType
typename PixelContainer::Pointer PixelContainerPointer
ImageBaseType::IndexType IndexType
PathConstIterator iterates (traces) over a path through an image.
typename TImage::PixelType PixelType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename TImage::InternalPixelType InternalPixelType
typename TImage::AccessorType AccessorType
typename TImage::OffsetType OffsetType
typename PathType::OutputType PathOutputType